Get Ahead in iOS Development: Grasp Core Location with Swift Today
When creating apps that require users’ location data—be it for showing a local weather forecast, tracking fitness routes, or recommending nearby restaurants—iOS developers need a reliable and efficient tool. Apple’s Core Location framework offers just that. With it, developers can integrate location services into their iOS apps with ease.
Table of Contents
In this blog post, we’ll delve into how to use Core Location with Swift to enhance your iOS applications. We’ll walk you through the basics and provide examples to help you get started.
1. Understanding Core Location
Core Location is a framework provided by Apple that allows applications to retrieve and monitor the device’s geographical location. It supports:
– Obtaining the device’s current location.
– Monitoring regions and getting notified when a user enters or exits a particular area.
– Monitoring the user’s location as they move.
– Determining the direction in which the device is moving.
2. Setting Up Core Location
Before you dive into code, you need to do some groundwork:
- Add Core Location to your project: Go to `File` -> `Add Packages…` in Xcode and search for Core Location.
- Add Privacy Descriptions in `Info.plist`:
– If you’re accessing the user’s location while the app is in the foreground, you need to add a description for the `NSLocationWhenInUseUsageDescription` key.
– For background location access, use `NSLocationAlwaysUsageDescription`.
3. Basic Implementation
Here’s a simple way to get the user’s current location:
- Import Core Location:
```swift import CoreLocation ```
- Conform to the `CLLocationManagerDelegate` protocol:
```swift class ViewController: UIViewController, CLLocationManagerDelegate { let locationManager = CLLocationManager() override func viewDidLoad() { super.viewDidLoad() locationManager.delegate = self locationManager.requestWhenInUseAuthorization() locationManager.requestLocation() } func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) { if let location = locations.last { print("Latitude: \(location.coordinate.latitude), Longitude: \(location.coordinate.longitude)") } } func locationManager(_ manager: CLLocationManager, didFailWithError error: Error) { print("Error: \(error)") } } ```
This basic setup initializes the location manager, requests authorization, and tries to fetch the current location.
4. Monitoring User Movement
To continuously track a user’s location:
```swift locationManager.startUpdatingLocation() ```
To stop:
```swift locationManager.stopUpdatingLocation() ```
5. Geofencing with Core Location
Geofencing is setting up a virtual boundary around a geographical area. With Core Location, you can monitor these boundaries and get notified when the user enters or exits these regions.
```swift let geofenceRegionCenter = CLLocationCoordinate2DMake(37.7833, -122.4167) let geofenceRegion = CLCircularRegion(center: geofenceRegionCenter, radius: 500, identifier: "SanFranciscoGeofence") geofenceRegion.notifyOnEntry = true geofenceRegion.notifyOnExit = true locationManager.startMonitoring(for: geofenceRegion) ```
To handle these events:
```swift func locationManager(_ manager: CLLocationManager, didEnterRegion region: CLRegion) { print("Entered \(region.identifier)") } func locationManager(_ manager: CLLocationManager, didExitRegion region: CLRegion) { print("Exited \(region.identifier)") } ```
6. Fetching Places with Reverse Geocoding
Core Location can translate latitude and longitude coordinates into human-readable places.
```swift func reverseGeocode(location: CLLocation) { let geocoder = CLGeocoder() geocoder.reverseGeocodeLocation(location) { (placemarks, error) in if let firstPlacemark = placemarks?.first { print(firstPlacemark.locality ?? "Location not found") } } } ```
7. Important Points to Remember
– Accuracy: You can set the desired accuracy of the location updates using `locationManager.desiredAccuracy`. However, higher accuracy can lead to faster battery drain.
– Request Permission: Always ensure you’re requesting the appropriate permission (`requestWhenInUseAuthorization()` or `requestAlwaysAuthorization()`) based on your app’s requirement.
– Check Location Services Availability: Use `CLLocationManager.locationServicesEnabled()` to check whether location services are enabled on the device.
Conclusion
Core Location offers iOS developers a powerful tool to enrich their applications with location-based features. With a basic understanding and the examples provided, you can integrate location services, track user movements, set up geofences, and translate coordinates into places. As with any tool that accesses sensitive user data, it’s imperative always to respect user privacy and permissions when using Core Location in your applications.
Table of Contents
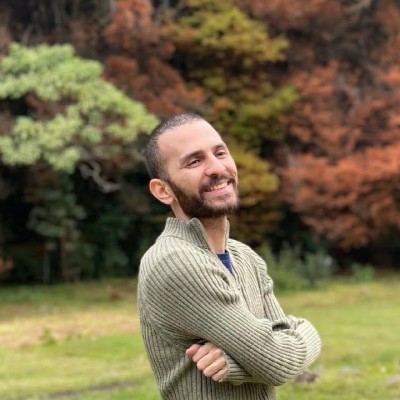
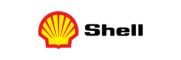