Crafting Motion-Driven Experiences: Swift & Core Motion Unveiled
The ability to detect and respond to device motion has unlocked new realms of user interaction and app experiences. From fitness trackers that count your steps to augmented reality games that immerse users in the real world, motion plays a pivotal role in these experiences. For those looking to harness this potential, it’s often beneficial to hire Swift developers. At the heart of many of these applications on iOS is Apple’s Core Motion framework.
Table of Contents
In this blog post, we will explore Core Motion, its potential, and see some practical examples of how it can be used in Swift-based iOS apps.
1. What is Core Motion?
Core Motion is an iOS framework that allows you to access and interpret the motion data from the device’s built-in sensors, including the accelerometer, gyroscope, magnetometer, and barometer.
2. Setting Up Core Motion
First, to work with Core Motion, you need to import it. Ensure you’ve added the necessary privacy descriptions in the app’s `Info.plist` if needed.
```swift import CoreMotion ```
Create an instance of `CMMotionManager`, which will act as your primary interface to the motion services.
```swift let motionManager = CMMotionManager() ```
Example 1: Detecting Device Orientation
With Core Motion, you can easily determine if a device is face up, face down, or in any other orientation. This can be particularly useful in applications where device orientation might change the user experience.
```swift if motionManager.isDeviceMotionAvailable { motionManager.deviceMotionUpdateInterval = 0.2 motionManager.startDeviceMotionUpdates(to: OperationQueue.current!) { (data, error) in if let data = data { if fabs(data.gravity.z) > 0.9 { print("Device is face up or face down") } else { print("Device is in another orientation") } } } } ```
Example 2: Step Counting
Imagine you’re developing a health application. By leveraging Core Motion’s pedometer data, you can count steps.
```swift let pedometer = CMPedometer() if CMPedometer.isStepCountingAvailable() { pedometer.startUpdates(from: Date()) { (data, error) in if let data = data { print("Steps taken: \(data.numberOfSteps)") } } } ```
Example 3: Gyroscope-based Animations
Gyroscope data can be used to animate objects based on device rotation. This is useful in applications like photo viewers, where images can be tilted based on device movements.
```swift if motionManager.isGyroAvailable { motionManager.gyroUpdateInterval = 0.2 motionManager.startGyroUpdates(to: OperationQueue.current!) { (data, error) in if let rotationRate = data?.rotationRate { // Use rotationRate.x, rotationRate.y, and rotationRate.z to animate objects. } } } ```
Example 4: Driving Game Controls
For game developers, Core Motion can provide intuitive controls. Imagine a car racing game where tilting the device controls the direction of the car.
3. Caveats and Recommendations
- Battery Usage: Continual motion data collection can drain the battery. It’s essential to stop updates when not needed.
- Check Availability: Always check if a particular feature (like step counting or gyroscope) is available on the device.
- Handling Errors: The examples above don’t handle errors for brevity. In real-world applications, ensure you handle potential errors appropriately.
- User Privacy: If collecting sensitive motion data, always inform the user and ensure you have the necessary permissions.
Conclusion
Core Motion provides a powerful toolset for iOS developers, making it an enticing reason to hire Swift developers. It unlocks a myriad of possibilities ranging from health applications to immersive gaming experiences. By understanding and harnessing this framework, developers can offer intuitive and interactive app experiences that feel natural and engaging to users. As you venture into these capabilities, always prioritize user privacy and provide transparency about how motion data is used in your applications.
Table of Contents
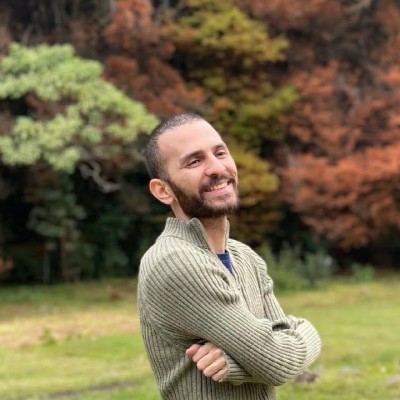
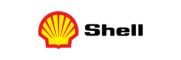