Swift and Core NFC: Incorporating Near Field Communication in iOS
Introduction to Near Field Communication (NFC)
Near Field Communication (NFC) is a short-range wireless technology that enables communication between devices when they are close to each other, typically within a few centimeters. NFC is widely used for contactless payments, access control, data exchange, and more. With Core NFC, iOS developers can now integrate NFC functionality into their apps, allowing users to read and interact with NFC tags.
Getting Started with Core NFC in Swift
Core NFC is the framework that Apple provides for reading NFC tags on iOS devices. It allows you to detect NFC tags, read their content, and trigger actions based on the data received. Below, we’ll explore how to set up a basic NFC reader using Swift.
1. Setting Up the Project
To begin, you need to ensure that your Xcode project is properly configured to use Core NFC. This involves enabling the NFC Tag Reading capability.
– Go to your project settings in Xcode.
– Select the target for your app.
– Under the “Signing & Capabilities” tab, click the “+” button to add a new capability.
– Search for “Near Field Communication Tag Reading” and add it.
Next, import the Core NFC framework in your Swift file:
```swift import CoreNFC ```
2. Implementing the NFC Reader
To implement an NFC reader, your view controller should conform to the `NFCNDEFReaderSessionDelegate` protocol. Here’s how to set up a basic NFC reading session:
```swift import UIKit import CoreNFC class NFCReaderViewController: UIViewController, NFCNDEFReaderSessionDelegate { var nfcSession: NFCNDEFReaderSession? override func viewDidLoad() { super.viewDidLoad() } @IBAction func startNFCSession(_ sender: Any) { nfcSession = NFCNDEFReaderSession(delegate: self, queue: nil, invalidateAfterFirstRead: false) nfcSession?.begin() } func readerSession(_ session: NFCNDEFReaderSession, didInvalidateWithError error: Error) { print("Session invalidated: \(error.localizedDescription)") } func readerSession(_ session: NFCNDEFReaderSession, didDetectNDEFs messages: [NFCNDEFMessage]) { for message in messages { for record in message.records { if let payload = String(data: record.payload, encoding: .utf8) { print("NFC Tag Detected: \(payload)") } } } } } ```
3. Reading Data from NFC Tags
The `didDetectNDEFs` method is called when an NFC tag is detected. Each detected tag contains one or more `NFCNDEFMessage` objects, which hold `NFCNDEFPayload` data. You can extract and process this data to perform actions based on the tag’s content.
For example, if the tag contains a URL, you might open it in a web view:
```swift func readerSession(_ session: NFCNDEFReaderSession, didDetectNDEFs messages: [NFCNDEFMessage]) { for message in messages { for record in message.records { if let url = record.wellKnownTypeURIPayload() { DispatchQueue.main.async { UIApplication.shared.open(url) } } } } } ```
4. Handling NFC Tag Types
Core NFC supports reading various types of NFC tags, including NDEF (NFC Data Exchange Format) tags. You can use the `NFCNDEFMessage` and `NFCNDEFPayload` classes to manage and interact with the data stored on these tags.
Example: Reading Text from an NFC Tag
```swift func readerSession(_ session: NFCNDEFReaderSession, didDetectNDEFs messages: [NFCNDEFMessage]) { for message in messages { for record in message.records { if let text = record.wellKnownTypeTextPayload().0 { print("NFC Tag Text: \(text)") } } } } ```
5. Writing Data to NFC Tags
While iOS primarily focuses on reading NFC tags, iOS 13 and later also support writing to certain types of NFC tags. This functionality can be used for creating tags that trigger specific actions when scanned.
Example: Writing an NDEF Message to a Tag
```swift func writeNFCMessage() { let urlPayload = NFCNDEFPayload.wellKnownTypeURIPayload(url: URL(string: "https://example.com")!) let message = NFCNDEFMessage(records: [urlPayload]) nfcSession?.writeNDEF(message, completionHandler: { (error) in if let error = error { print("Write failed: \(error.localizedDescription)") } else { print("Write successful") } }) } ```
Conclusion
Incorporating NFC functionality into your iOS app using Swift and Core NFC opens up a world of possibilities for enhancing user interactions. Whether you’re building a payment system, an access control app, or simply want to read NFC tags for data exchange, Swift provides a powerful and flexible environment for leveraging NFC technology.
Further Reading:
Table of Contents
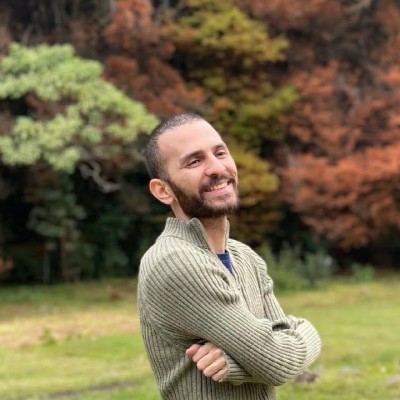
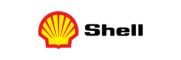