Swift and Core Spotlight: Making iOS App Content Discoverable
Making content within an iOS app easily discoverable is crucial for enhancing user experience and engagement. Core Spotlight, an Apple framework, allows developers to index app content and make it searchable using the iOS Spotlight search feature. This blog explores how to leverage Swift and Core Spotlight to make your iOS app’s content more discoverable and provide practical examples for implementation.
Understanding Core Spotlight
Core Spotlight is a framework provided by Apple that enables iOS apps to integrate their content into the system-wide Spotlight search. By indexing app content with Core Spotlight, users can find relevant information directly from the search bar, making it easier to access and interact with your app’s data.
Using Swift to Integrate Core Spotlight
Swift, with its modern syntax and robust libraries, is well-suited for implementing Core Spotlight functionality in iOS apps. Below are key aspects and code examples demonstrating how Swift can be used to make app content discoverable with Core Spotlight.
1. Indexing Content with Core Spotlight
To make content discoverable, you need to index it using Core Spotlight. This involves creating `CSSearchableItem` objects and adding them to the search index.
Example: Indexing App Content
Here’s how you can index an article in your app using Core Spotlight:
```swift import CoreSpotlight import MobileCoreServices func indexArticle(title: String, content: String, identifier: String) { let searchableItemAttributeSet = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) searchableItemAttributeSet.title = title searchableItemAttributeSet.contentDescription = content let searchableItem = CSSearchableItem(uniqueIdentifier: identifier, domainIdentifier: nil, attributeSet: searchableItemAttributeSet) CSSearchableIndex.default().indexSearchableItems([searchableItem]) { (error) in if let error = error { print("Error indexing item: \(error.localizedDescription)") } else { print("Item indexed successfully.") } } } ```
2. Updating and Deleting Indexed Content
If the content in your app changes, you need to update the existing index or remove it if necessary.
Example: Updating Indexed Content
```swift func updateArticle(identifier: String, newTitle: String, newContent: String) { let searchableItemAttributeSet = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) searchableItemAttributeSet.title = newTitle searchableItemAttributeSet.contentDescription = newContent let searchableItem = CSSearchableItem(uniqueIdentifier: identifier, domainIdentifier: nil, attributeSet: searchableItemAttributeSet) CSSearchableIndex.default().indexSearchableItems([searchableItem]) { (error) in if let error = error { print("Error updating item: \(error.localizedDescription)") } else { print("Item updated successfully.") } } } ```
Example: Deleting Indexed Content
```swift func deleteArticle(identifier: String) { CSSearchableIndex.default().deleteSearchableItems(withIdentifiers: [identifier]) { (error) in if let error = error { print("Error deleting item: \(error.localizedDescription)") } else { print("Item deleted successfully.") } } } ```
3. Handling Search Results
When users search for indexed content, they can be directed to specific content within your app. You need to handle these search results to provide a seamless user experience.
Example: Handling Search Results
```swift import CoreSpotlight import MobileCoreServices func handleSearchResults(userInfo: [String: Any]) { if let userInfo = userInfo[CSSearchableItemActionType] as? [String: Any], let identifier = userInfo[CSSearchableItemIdentifierKey] as? String { // Navigate to the content based on the identifier print("User tapped on item with identifier: \(identifier)") // Example: Navigate to the relevant screen } } ```
4. Customizing the Search Experience
You can further customize the search experience by using advanced features of Core Spotlight, such as domain identifiers and custom metadata.
Example: Adding Domain Identifiers
```swift func indexArticleWithDomain(identifier: String, title: String, content: String, domain: String) { let searchableItemAttributeSet = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) searchableItemAttributeSet.title = title searchableItemAttributeSet.contentDescription = content let searchableItem = CSSearchableItem(uniqueIdentifier: identifier, domainIdentifier: domain, attributeSet: searchableItemAttributeSet) CSSearchableIndex.default().indexSearchableItems([searchableItem]) { (error) in if let error = error { print("Error indexing item: \(error.localizedDescription)") } else { print("Item indexed successfully with domain.") } } } ```
Conclusion
Swift and Core Spotlight offer powerful tools for making your iOS app’s content discoverable. By indexing, updating, and handling search results effectively, you can enhance user experience and ensure that valuable content is easily accessible. Implementing Core Spotlight in your app will contribute to a more engaging and user-friendly experience.
Further Reading:
Table of Contents
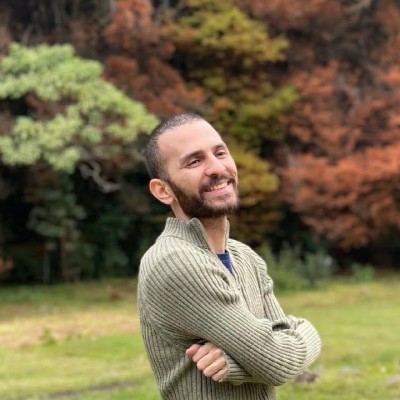
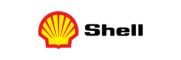