Swift and Core Telephony: Building Telephony Features into iOS Apps
Core Telephony is a framework provided by Apple that allows developers to access telephony-related information on iOS devices. This includes data such as carrier information, call states, and cellular network details. By integrating Core Telephony into your iOS apps, you can build features that enhance the user experience by leveraging the device’s telephony capabilities.
This article explores how Swift, Apple’s powerful and intuitive programming language, can be used in conjunction with Core Telephony to create telephony-enabled iOS applications.
Setting Up Core Telephony in Swift
Before diving into code examples, itβs essential to understand how to set up Core Telephony in your Swift project. You need to import the `CoreTelephony` framework and ensure your app has the necessary permissions.
Example: Importing Core Telephony and Setting Permissions
```swift import CoreTelephony let networkInfo = CTTelephonyNetworkInfo() let carrier = networkInfo.serviceSubscriberCellularProviders ```
You also need to update your app’s `Info.plist` to request permission to access telephony information:
```xml <key>NSTelephonyUsageDescription</key> <string>This app requires access to telephony information.</string> ```
1. Accessing Carrier Information
One of the primary uses of Core Telephony is to access carrier-related information such as the carrier name, mobile country code, and mobile network code. This data can be used to tailor the app’s behavior based on the user’s network provider.
Example: Fetching Carrier Information
```swift import CoreTelephony let networkInfo = CTTelephonyNetworkInfo() if let carrier = networkInfo.subscriberCellularProvider { print("Carrier Name: \(carrier.carrierName ?? "Unknown")") print("Mobile Country Code: \(carrier.mobileCountryCode ?? "Unknown")") print("Mobile Network Code: \(carrier.mobileNetworkCode ?? "Unknown")") } ```
2. Monitoring Call States
Core Telephony also enables developers to monitor the call state of the device. This can be useful in apps where you want to pause certain activities when a call is in progress or detect when a call ends to resume those activities.
Example: Monitoring Call States
```swift import CoreTelephony let callCenter = CTCallCenter() callCenter.callEventHandler = { call in switch call.callState { case CTCallStateDialing: print("Dialing") case CTCallStateIncoming: print("Incoming Call") case CTCallStateConnected: print("Call Connected") case CTCallStateDisconnected: print("Call Disconnected") default: print("Unknown Call State") } } ```
3. Handling Cellular Network Information
You can also access cellular network information using Core Telephony. This data can be valuable for apps that need to adapt based on the network type, such as optimizing content delivery over LTE or 5G.
Example: Accessing Cellular Network Information
```swift import CoreTelephony let networkInfo = CTTelephonyNetworkInfo() if let radioAccessTechnology = networkInfo.serviceCurrentRadioAccessTechnology { for (key, value) in radioAccessTechnology { print("Radio Access Technology for \(key): \(value)") } } ```
4. Integrating with Other Telephony Features
In addition to the basics, you can combine Core Telephony with other frameworks or services to build more complex telephony features. For example, you can integrate with `CallKit` to provide a native calling experience or `PushKit` to handle VoIP calls more efficiently.
Example: Using Core Telephony with CallKit
```swift import CallKit let callController = CXCallController() let handle = CXHandle(type: .phoneNumber, value: "1234567890") let startCallAction = CXStartCallAction(call: UUID(), handle: handle) let transaction = CXTransaction(action: startCallAction) callController.request(transaction) { error in if let error = error { print("Error requesting transaction: \(error.localizedDescription)") } else { print("Call started successfully") } } ```
Conclusion
Swift and Core Telephony provide powerful tools to build telephony features into your iOS apps. Whether you’re accessing carrier information, monitoring call states, or integrating with other telephony-related frameworks, these capabilities can significantly enhance the functionality and user experience of your app.
Leveraging Core Telephony effectively requires careful consideration of privacy and permissions, but the result is a more responsive and tailored application for your users.
Further Reading:
Table of Contents
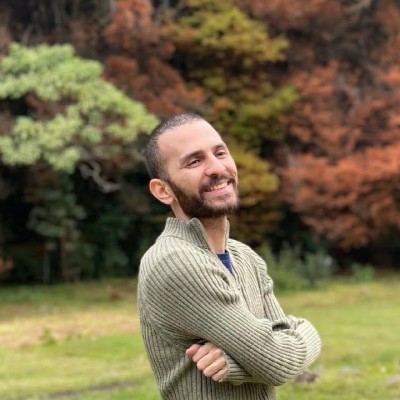
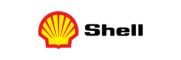