Building Smarter iOS Apps: Integrating Machine Learning with Swift and CoreML
With the advent of Machine Learning (ML) and Artificial Intelligence (AI), the tech world has seen a paradigm shift in what can be accomplished using data and complex algorithms. These advancements have made a significant impact on mobile app development, leading to a rise in demand to hire Swift developers who can utilize ML models to provide sophisticated features like facial recognition, sentiment analysis, and image classification, among others. This article focuses on how we can integrate machine learning models into iOS apps using Swift and CoreML, an essential skill for any Swift developers you might be looking to hire.
Table of Contents
1. Introduction to CoreML
Core ML is a framework provided by Apple that helps developers integrate machine learning models into their apps. The great advantage of CoreML is that it allows developers to use machine learning models without requiring a deep understanding of machine learning or AI concepts. CoreML supports a variety of model types, including neural networks, tree ensembles, support vector machines, and generalized linear models.
2. Converting ML Models for CoreML
Before integrating into an iOS app, the machine learning models need to be converted into Core ML compatible format (.mlmodel). Apple provides a Python package, coremltools, for this conversion. It supports popular models from Keras, Scikit-learn, XGBoost, LibSVM, and more.
For instance, if we have a Keras model (let’s say `my_model.h5`), the conversion can be done with a few lines of Python code:
```python import coremltools # Load the Keras model keras_model = load_model('my_model.h5') # Convert the model coreml_model = coremltools.converters.keras.convert(keras_model) # Save the converted model coreml_model.save('MyCoreMLModel.mlmodel') ```
After conversion, the .mlmodel file can be directly imported into an Xcode project.
3. Importing the Model into Xcode
After you have the .mlmodel file, you can import it into your Xcode project by dragging and dropping the file into the project navigator. Xcode will automatically generate an interface to the model that you can use in Swift.
4. Using the Model in Swift
Once the .mlmodel file is included in your Xcode project, it’s straightforward to use the model to make predictions. Suppose we have a sentiment analysis model that predicts the sentiment of a piece of text. Here’s an example of how you might use this model in Swift:
```swift import CoreML // Assume we have a model named `SentimentClassifier` let model = try? SentimentClassifier(configuration: MLModelConfiguration()) let input = SentimentClassifierInput(text: "I love this app!") let prediction = try? model?.prediction(input: input) // Print out the predicted sentiment if let sentiment = prediction?.label { print("Predicted sentiment: \(sentiment)") } ```
In this example, `SentimentClassifier` is the Swift class automatically generated by Xcode when you import the .mlmodel file. We create an instance of this class and then use its `prediction` method to make a prediction.
5. Real-World Example: Image Classification
To give you a clearer picture of the process, let’s dive into a real-world example using an image classification model. We’ll use the MobileNetV2 model, which has been trained to classify images into 1,000 different categories.
5.1. Convert the Model to CoreML Format
You can download the MobileNetV2 model in Keras format from the Keras Applications page. To convert it to CoreML format, we’ll use coremltools:
```python import coremltools from keras.applications import MobileNetV2 # Load the Keras model keras_model = MobileNetV2(weights="imagenet") # Convert the model coreml_model = coremltools.converters.keras.convert(keras_model, input_names=['image'], image_input_names='image', output_names=['classLabelProbs', 'classLabel'], class_labels='labels.txt') # Save the converted model coreml_model.save('MobileNetV2.mlmodel') ```
5.2. Import the Model into Xcode
Like before, you can import the MobileNetV2.mlmodel file into your Xcode project by dragging and dropping.
5.3. Use the Model in Swift
Here’s how you can use the MobileNetV2 model to classify an image:
```swift import CoreML import Vision // Create a Vision Core ML model from our Core ML model let coreMLModel = try? VNCoreMLModel(for: MobileNetV2().model) let request = VNCoreMLRequest(model: coreMLModel!) { (request, error) in if let error = error { print("Error: \(error)") } else if let results = request.results as? [VNClassificationObservation] { // Print the 3 classifications with the highest confidence for result in results.prefix(3) { print("\(result.identifier): \(result.confidence)") } } } // Create a handler and perform the request let handler = VNImageRequestHandler(cgImage: myImage) try? handler.perform([request]) ```
In this example, we use the Vision framework to handle the image preprocessing required by the MobileNetV2 model. `VNCoreMLRequest` performs the model prediction, and then we print out the three most likely classifications.
Conclusion
Integrating CoreML with Swift offers numerous possibilities in creating intelligent iOS applications. These powerful tools allow developers, including those you might hire as Swift developers, to leverage complex machine learning models in their apps, providing users with advanced features such as image recognition and sentiment analysis.
With Apple’s CoreML, the process of incorporating these models has become streamlined and user-friendly, significantly lowering the barrier of entry for developers. Whether you’re considering hiring Swift developers to add a straightforward model to an existing app or to build an AI-centric app from the ground up, CoreML and Swift make the process not just possible, but easy and efficient.
Table of Contents
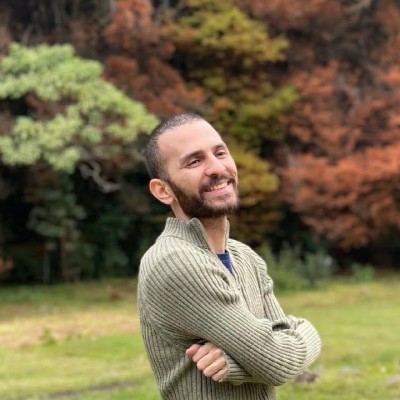
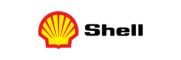