Swift Q & A
How do I create custom operators in Swift?
In Swift, you can create custom operators to extend the language’s capabilities and provide a more expressive and concise syntax for specific tasks. Custom operators allow you to define your own symbols, like `+`, `-`, `*`, and `/`, and specify their behavior when applied to your custom types or existing types. Here’s how you can create custom operators in Swift:
- Choose an Operator Symbol: To create a custom operator, choose a symbol that is not already defined in Swift. Custom operators can consist of a sequence of certain characters, including: `+`, `-`, `*`, `/`, `%`, `=`, `<`, `>`, `!`, `&`, `|`, `^`, `.`, and `~`. You can also use Unicode characters.
- Define Operator Functions: Create functions with the `prefix`, `infix`, or `postfix` keyword, depending on where you want to place your custom operator in relation to its operands. For example, if you want to create an infix operator “ for exponentiation, define a function with the `infix` keyword:
```swift infix operator : MultiplicationPrecedence func (base: Double, exponent: Double) -> Double { return pow(base, exponent) } ```
- Specify Operator Precedence: You should specify the precedence and associativity of your custom operator using operator precedence groups. In the example above, we use `MultiplicationPrecedence` to indicate that our operator has the same precedence as multiplication.
- Implement Operator Functionality: Inside your operator function, define the behavior of your custom operator when applied to its operands. In the example, the “ operator calculates exponentiation using the `pow` function.
- Use Your Custom Operator: Once defined, you can use your custom operator like any built-in operator. For instance:
```swift let result = 2.0 3.0 // This calculates 2.0 raised to the power of 3.0 ```
Custom operators can be a powerful tool when used thoughtfully and sparingly in your codebase. However, it’s essential to provide clear documentation and ensure that your custom operators enhance code readability and maintainability rather than making it more cryptic or confusing.
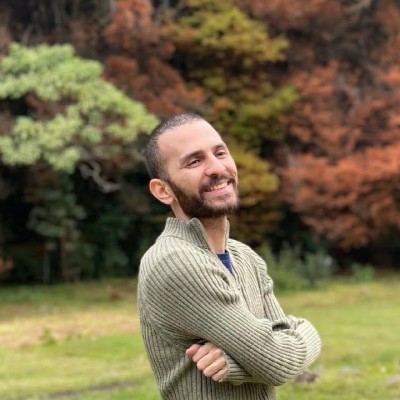
Previously at
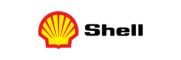
Experienced iOS Engineer with 7+ years mastering Swift. Created fintech solutions, enhanced biopharma apps, and transformed retail experiences.