How do I create custom protocols in Swift?
Creating custom protocols in Swift is a fundamental aspect of protocol-oriented programming, allowing you to define a blueprint for methods, properties, and functionalities that can be adopted by classes, structs, or enums. Custom protocols enable you to establish a contract for conforming types, ensuring that they implement specific behaviors. Here’s a detailed guide on how to create custom protocols in Swift:
- Define a Protocol: To create a custom protocol, you start by using the `protocol` keyword, followed by the name of your protocol. Inside the protocol, you specify the methods, properties, or associated types that conforming types must implement. Here’s a basic example of a custom protocol called `Drawable`:
```swift protocol Drawable { func draw() } ```
- Conforming to a Protocol: Once you’ve defined your custom protocol, you can make classes, structs, or enums conform to it by implementing its requirements. Conforming types must provide concrete implementations for all the methods and properties declared in the protocol. For instance:
```swift struct Circle: Drawable { func draw() { // Implement drawing logic for a circle } } ```
- Multiple Protocol Conformance: Swift allows a type to conform to multiple protocols. This is a powerful feature that enables you to define more complex behaviors by combining multiple protocols. For example:
```swift protocol Rotatable { func rotate() } struct Square: Drawable, Rotatable { func draw() { // Implement drawing logic for a square } func rotate() { // Implement rotation logic for a square } } ```
- Protocol Inheritance: Protocols in Swift can also inherit from other protocols. This allows you to build on existing protocols and define more specialized ones. For example:
```swift protocol Printable { func printDescription() } protocol DrawableAndPrintable: Drawable, Printable { // Inherits the requirements from both Drawable and Printable } ```
- Using Protocol Extensions: You can provide default implementations for methods in a protocol using protocol extensions. This allows you to offer a common implementation that conforming types can choose to override.
```swift extension Drawable { func draw() { // Default implementation for drawing } } ```
In conclusion, creating custom protocols in Swift is a versatile way to define a contract for conforming types, enabling you to build modular and extensible code. Whether you need to establish common behavior for different types or create more specialized protocols, custom protocols are a fundamental concept in Swift’s protocol-oriented programming paradigm.
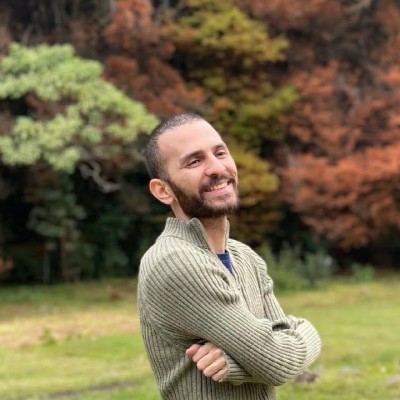
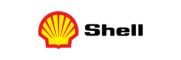