Data Persistence in Swift: Storing and Retrieving Data in iOS Apps
Data persistence is a crucial aspect of iOS app development, allowing you to store and retrieve data even when the app is closed or the device is restarted. Whether you need to save user preferences, cache data, or manage user-generated content, implementing data persistence is essential for a seamless user experience. In this blog post, we will explore various techniques and code samples to help you master data persistence in Swift for iOS apps.
Why Data Persistence Matters in iOS Apps
Data persistence allows you to preserve data across app launches, device reboots, and even updates. Without data persistence, your app would lose all its stored data, leading to a poor user experience. Whether it’s user preferences, cached data, or offline content, the ability to store and retrieve data efficiently is vital for app functionality.
Introduction to Data Persistence Techniques
There are several techniques available for implementing data persistence in iOS apps. Let’s explore some of the commonly used ones:
1. UserDefaults
UserDefaults is a lightweight and straightforward way to store small amounts of data, such as user preferences and settings. It works based on key-value pairs and offers a simple interface for storing and retrieving data. However, UserDefaults is not suitable for storing large amounts of complex data.
2. Property Lists
Property lists, also known as plists, are XML or binary files that can store various types of data, including arrays, dictionaries, and primitive types. Property lists are easy to use and can handle moderate amounts of data. They are a good choice for managing app configurations or storing structured data.
3. SQLite Databases
SQLite is a widely used open-source relational database engine that can be integrated into iOS apps. It provides a powerful and flexible way to store, query, and manage structured data. SQLite databases are suitable for handling large amounts of data and complex data relationships.
4. Core Data
Core Data is a framework provided by Apple for managing object graphs and persisting data in iOS and macOS apps. It offers a high-level abstraction layer over SQLite databases, making it easier to work with data models, relationships, and querying. Core Data is ideal for handling complex data models and relationships.
5. Realm
Realm is a mobile database solution that provides an alternative to SQLite and Core Data. It offers an object-oriented approach to data persistence and supports real-time data synchronization. Realm is known for its simplicity, speed, and cross-platform compatibility.
Implementing Data Persistence Techniques in Swift
Now let’s dive into implementing each of these data persistence techniques using Swift. We’ll provide code samples to demonstrate how to store and retrieve data using each approach.
1. UserDefaults Example
swift // Storing a value UserDefaults.standard.set("John Doe", forKey: "username") // Retrieving a value if let username = UserDefaults.standard.string(forKey: "username") { print("Username: \(username)") } else { print("Username not found") }
2. Property Lists Example
swift // Storing data let data = ["apple", "banana", "orange"] let plistURL = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask).first?.appendingPathComponent("fruits.plist") (data as NSArray).write(to: plistURL, atomically: true) // Retrieving data if let loadedData = NSArray(contentsOf: plistURL) as? [String] { print("Loaded data: \(loadedData)") } else { print("Failed to load data") }
3. SQLite Databases Example
swift // Setting up the database let fileURL = try! FileManager.default.url(for: .documentDirectory, in: .userDomainMask, appropriateFor: nil, create: false) .appendingPathComponent("app.db") let database = FMDatabase(url: fileURL) database.open() // Creating a table let createTableQuery = "CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY AUTOINCREMENT, name TEXT, email TEXT)" database.executeStatements(createTableQuery) // Inserting data let insertQuery = "INSERT INTO users (name, email) VALUES (?, ?)" database.executeUpdate(insertQuery, withArgumentsIn: ["John Doe", "john.doe@example.com"]) // Retrieving data let selectQuery = "SELECT * FROM users" if let resultSet = database.executeQuery(selectQuery, withArgumentsIn: []) { while resultSet.next() { let name = resultSet.string(forColumn: "name") ?? "" let email = resultSet.string(forColumn: "email") ?? "" print("Name: \(name), Email: \(email)") } } else { print("Failed to execute query") } database.close()
4. Core Data Example
swift // Defining a Core Data model class User: NSManagedObject { @NSManaged var name: String? @NSManaged var email: String? } // Saving data let context = persistentContainer.viewContext let user = User(context: context) user.name = "John Doe" user.email = "john.doe@example.com" do { try context.save() } catch { print("Failed to save data: \(error)") } // Fetching data let fetchRequest: NSFetchRequest<User> = User.fetchRequest() if let fetchedUsers = try? context.fetch(fetchRequest) { for user in fetchedUsers { if let name = user.name, let email = user.email { print("Name: \(name), Email: \(email)") } } } else { print("Failed to fetch data") }
5. Realm Example
swift // Defining a Realm model class User: Object { @Persisted var name: String? @Persisted var email: String? } // Configuring and opening a Realm instance let configuration = Realm.Configuration.defaultConfiguration let realm = try! Realm(configuration: configuration) // Writing data try! realm.write { let user = User() user.name = "John Doe" user.email = "john.doe@example.com" realm.add(user) } // Retrieving data let users = realm.objects(User.self) for user in users { if let name = user.name, let email = user.email { print("Name: \(name), Email: \(email)") } }
Choosing the Right Data Persistence Technique
When deciding which data persistence technique to use in your iOS app, consider factors such as the type and amount of data, performance requirements, and complexity of the data model. UserDefaults and Property Lists are suitable for small amounts of simple data, while SQLite, Core Data, and Realm excel at handling larger data sets and complex relationships.
Best Practices for Data Persistence in Swift
- Understand the data persistence technique you choose and its limitations.
- Optimize data storage by choosing the appropriate data structure and serialization format.
- Separate concerns by using data models or objects dedicated to data persistence.
- Handle data migrations carefully when modifying your app’s data model.
- Test and verify your data persistence implementation thoroughly.
Conclusion
Implementing data persistence in iOS apps is crucial for maintaining app state and providing a seamless user experience. In this blog post, we explored various data persistence techniques in Swift, including UserDefaults, Property Lists, SQLite Databases, Core Data, and Realm. By understanding these techniques and leveraging code samples, you can choose the right approach for your app and effectively store and retrieve data. Remember to follow best practices and test your implementation to ensure reliable and efficient data persistence in your iOS apps.
Table of Contents
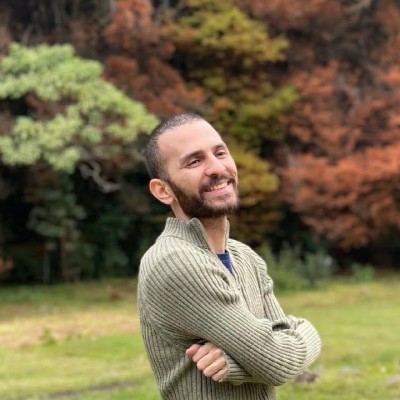
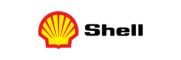