How do I work with dates and times in Swift?
Working with dates and times in Swift is essential for many applications, whether you’re dealing with scheduling, displaying timestamps, or performing date calculations. Swift provides the `Date` structure and various supporting classes in the Foundation framework to handle date and time operations effectively.
Here’s how you can work with dates and times in Swift:
- Creating Dates:
You can create a `Date` instance representing the current date and time using `Date()` or specify a specific date and time using `DateComponents` and `Calendar`. For example:
```swift let currentDate = Date() let calendar = Calendar.current var dateComponents = DateComponents() dateComponents.year = 2023 dateComponents.month = 5 dateComponents.day = 15 let customDate = calendar.date(from: dateComponents) ```
- Formatting and Displaying Dates:
You can format `Date` instances into human-readable strings using `DateFormatter`. This allows you to display dates in various styles and formats:
```swift let formatter = DateFormatter() formatter.dateFormat = "MMM dd, yyyy HH:mm" let formattedDate = formatter.string(from: currentDate) ```
- Date Calculations:
You can perform calculations on dates, such as adding or subtracting time intervals, comparing dates, and finding the time difference between them. Swift provides methods and properties for these operations:
```swift let tomorrow = Calendar.current.date(byAdding: .day, value: 1, to: currentDate) let timeDifference = tomorrow!.timeIntervalSince(currentDate) ```
- Working with Time Zones:
When working with dates and times, consider time zones. You can set the time zone when creating a `DateFormatter` or convert dates between time zones using `TimeZone` and `Calendar`.
- Date Components:
You can extract specific components like year, month, day, hour, minute, and second from a `Date` using `Calendar` and `DateComponents`.
- Handling Time Intervals:
Swift provides `TimeInterval` to represent time intervals in seconds. You can use this for timeouts, scheduling, and duration calculations.
Working with dates and times in Swift requires a good understanding of the `Date` structure, `DateFormatter`, `Calendar`, and other related classes. It’s essential to consider time zones, daylight saving time, and other factors to ensure accurate and reliable date and time operations in your applications.
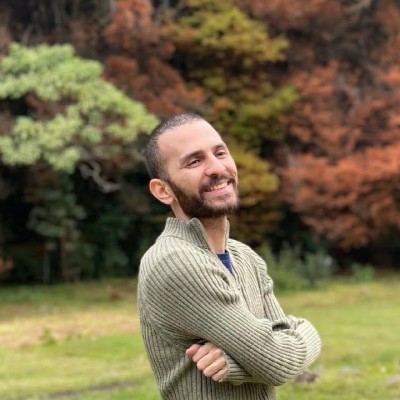
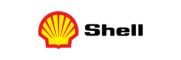