What is the purpose of ‘defer’ in Swift?
In Swift, the `defer` statement is a powerful tool for managing the execution flow of code blocks, particularly when it comes to resource cleanup, ensuring that certain tasks are executed regardless of how a function or code block exits. The primary purpose of `defer` is to postpone the execution of a block of code until the current scope exits, whether that’s due to a return statement, an error, or just the natural flow of the program.
Here’s how `defer` works and its key purposes:
- Resource Cleanup: One of the most common uses of `defer` is for resource cleanup. For example, if you open a file, allocate memory, or acquire a lock, you want to ensure that these resources are properly released, regardless of how the function exits. With `defer`, you can place the cleanup code at the beginning of the function, and it will be executed when the function returns or exits, ensuring proper resource management.
- Reversing Actions: `defer` is also helpful when you need to reverse actions that were performed earlier in the code. For example, if you modify some data or settings within a function and want to undo those changes before exiting, you can use `defer` to reverse those actions.
- Maintaining Clean Code: `defer` promotes clean and readable code by allowing you to keep related operations together. Instead of scattering cleanup code throughout a function, you can place it all in one `defer` block at the beginning of the function, making it clear and easy to understand.
Here’s a simple example illustrating the use of `defer` for resource cleanup:
```swift func processFile() { let file = openFile() defer { closeFile(file) } // Code to read and process the file goes here // 'file' will be closed when this function exits, even if it's due to an error. } ```
In this example, the `closeFile()` function is deferred, ensuring that the file is closed when the function exits, regardless of the exit point.
Overall, `defer` is a valuable feature in Swift that promotes good coding practices by helping you maintain clean, organized, and reliable code, especially when it comes to resource management and reversing actions.
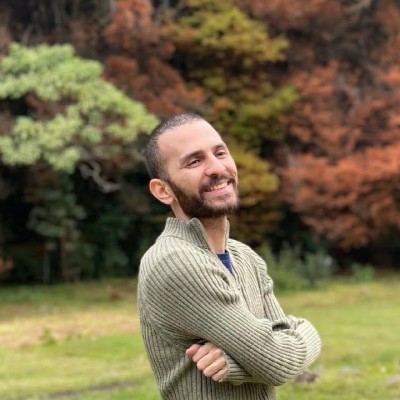
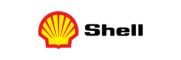