What is a delegate in Swift?
In Swift, a delegate is a design pattern that allows one object to act on behalf of, or in coordination with, another object. Delegates are commonly used for implementing the delegation pattern, which promotes loose coupling and separation of concerns in your code. The primary purpose of delegates is to delegate responsibilities and actions from one object (the delegating object) to another object (the delegate).
Key points about delegates in Swift:
- Delegate Protocol: Typically, the delegate pattern involves defining a delegate protocol that outlines a set of methods and optional methods that the delegate can implement. These methods represent events or actions that the delegating object can trigger.
- Conforming to the Protocol: Any object that wishes to act as a delegate must conform to the delegate protocol by implementing the required methods. This allows the delegate to respond to events and perform tasks defined by the delegating object.
- Loose Coupling: Delegates enable loose coupling between objects, as the delegating object does not need to know the specific class of its delegate. This promotes modularity and maintainability.
- Delegate Assignment: To establish the delegate relationship, you set the delegate property of the delegating object to the instance of an object that conforms to the delegate protocol. This connection allows the delegating object to communicate with the delegate.
- Common Use Cases: Delegates are commonly used in iOS and macOS development for tasks such as handling user interface events (e.g., responding to button taps), data source management (e.g., populating table views), and customizing behavior (e.g., text field validation).
Here’s a simplified example in Swift:
```swift protocol MyDelegate { func didPerformAction() } class DelegatingObject { var delegate: MyDelegate? func performAction() { // Perform some action delegate?.didPerformAction() } } class MyDelegateObject: MyDelegate { func didPerformAction() { print("Delegate object received the action.") } } let delegatingObj = DelegatingObject() let delegateObj = MyDelegateObject() delegatingObj.delegate = delegateObj delegatingObj.performAction() // Output: "Delegate object received the action." ```
In this example, `DelegatingObject` has a delegate of type `MyDelegate`, and `MyDelegateObject` conforms to the `MyDelegate` protocol. When `performAction()` is called on `delegatingObj`, it triggers the `didPerformAction()` method on the delegate, leading to the printed output.
Delegates are a powerful mechanism in Swift for achieving separation of concerns, enabling customizability, and promoting maintainable and modular code. They play a crucial role in many aspects of iOS, macOS, and watchOS development.
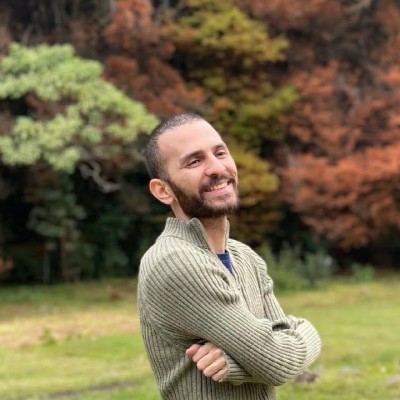
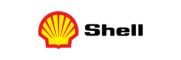