Elevate Your Swift Apps: Dependency Injection for the Modern Developer
Dependency Injection (DI) is a powerful design pattern, especially in the realm of iOS development. As businesses hire Swift developers, it’s crucial they understand the significance of maintaining clean, modular, and testable code. In essence, DI is a technique to supply an object with its dependencies, rather than having it construct them internally. This approach not only promotes flexibility and testability but also ensures scalability in applications.
Table of Contents
In this article, we’ll delve into the why and how of Dependency Injection in Swift, complete with practical examples.
1. Why Use Dependency Injection?
Before we explore the techniques, let’s understand why you might want to use DI:
- Testability: With DI, you can inject mock dependencies for testing, thus allowing unit tests to be independent of external systems.
- Code Reusability: By injecting dependencies, you decouple the logic from specific classes or structs, making code more reusable.
- Flexibility: Changing or swapping out parts of the system becomes easier, since dependencies are not hardcoded.
2. Types of Dependency Injection
There are primarily three types:
- Constructor Injection: Injecting the dependencies through the constructor (or initializer in Swift).
- Property Injection: Setting a property on an instance after it’s created.
- Method Injection: Providing the dependency using a method.
2.1 Examples of Dependency Injection in Swift
Let’s illustrate these concepts with Swift code:
- Constructor Injection
This is the most common form of DI. Here, the dependent objects are provided to the instance at the time of its creation.
```swift protocol Database { func fetchData() -> String } class SQLite: Database { func fetchData() -> String { return "Data from SQLite" } } class Service { let db: Database init(database: Database) { self.db = database } func retrieveData() -> String { return db.fetchData() } } let sqlite = SQLite() let service = Service(database: sqlite) print(service.retrieveData()) // Outputs: "Data from SQLite" ```
- Property Injection
Here, the dependency is set as a property of the instance after it has been initialized.
```swift class Service { var db: Database? func retrieveData() -> String { return db?.fetchData() ?? "No Data" } } let service = Service() let sqlite = SQLite() service.db = sqlite print(service.retrieveData()) // Outputs: "Data from SQLite" ```
Note: Use this approach with caution, as there’s the potential risk of accessing the property before it’s been set.
- Method Injection
In this method, the dependencies are passed as arguments to methods.
```swift class Service { func retrieveData(using db: Database) -> String { return db.fetchData() } } let service = Service() let sqlite = SQLite() print(service.retrieveData(using: sqlite)) // Outputs: "Data from SQLite" ```
3. Using Frameworks for Dependency Injection
While manual DI is straightforward for smaller projects, as your project grows, using a framework might be beneficial. Here’s a look at how to achieve DI using a popular framework named `Swinject`.
Install `Swinject` through CocoaPods, Carthage, or Swift Package Manager. Once integrated:
```swift import Swinject // Define your protocols and classes as before let container = Container() container.register(Database.self) { _ in SQLite() } container.register(Service.self) { resolver in Service(database: resolver.resolve(Database.self)!) } let service = container.resolve(Service.self)! print(service.retrieveData()) // Outputs: "Data from SQLite" ```
4. Best Practices
- Avoid Singleton Anti-Pattern: While singletons are tempting, they tightly couple code, making testing and refactoring difficult. If you must use a singleton, inject it rather than accessing it globally.
- Use Protocols: As seen in our examples, always code against abstractions (protocols in Swift) rather than concrete implementations. This makes it easier to swap, mock, or extend functionalities.
- Keep Configurations Centralized: If using a DI container/framework, centralize the configurations. It ensures that the system setup is coherent and understandable.
Conclusion
Dependency Injection isn’t just a fancy term. It’s a practical, beneficial design pattern that aids in producing robust, testable, and maintainable code. For businesses looking to hire Swift developers, it’s essential to note that while Swift doesn’t have built-in DI features like some languages, its emphasis on protocols and the existence of powerful DI frameworks makes it seamless to adopt this practice. As with any tool, the key is to understand when and how to use it effectively. Happy coding!
Table of Contents
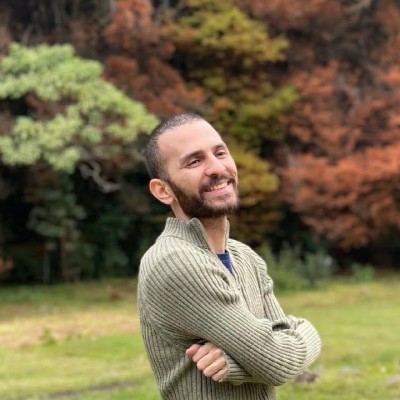
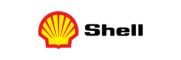