What is a didSet in Swift?
In Swift, `didSet` is a property observer that allows you to execute custom code immediately after the value of a property has been updated or set. This observer is particularly useful when you need to respond to changes in a property’s value, perform additional actions, or trigger side effects after a property has been modified.
Key points about `didSet` in Swift:
- Syntax: To use `didSet`, you declare it within the property’s scope, typically just below the property declaration. The observer is triggered automatically whenever the property’s value changes, whether the change occurs within the same type or externally.
- Access to oldValue: Within the `didSet` observer block, you have access to a special variable called `oldValue`, which holds the previous value of the property before it was updated. This allows you to compare the new value with the old value and perform actions based on the change.
- Common Use Cases: `didSet` is often used for tasks such as logging property changes, updating user interfaces to reflect property updates, or enforcing specific behaviors when a property’s value changes.
Here’s a simple example of `didSet` in Swift:
```swift var age: Int = 30 { didSet { if age < 0 { print("Age cannot be negative. Resetting to 0.") age = 0 } else if age > 120 { print("Age cannot exceed 120. Resetting to 120.") age = 120 } } } ```
In this example, the `didSet` observer for the `age` property ensures that the age is within a valid range (0 to 120) and resets it if it falls outside that range.
Overall, `didSet` is a valuable tool in Swift for maintaining data integrity, responding to property changes, and adding custom behavior when properties are modified, making it a powerful feature for property management and observation.
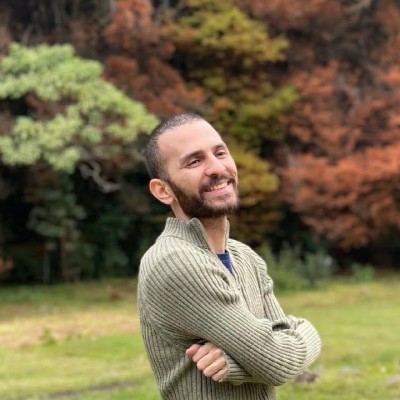
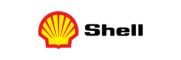