What is a didSet property observer in Swift?
In Swift, a `didSet` property observer is a feature that allows you to observe and respond to changes in the value of a property. It is a part of Swift’s property observers mechanism, and it provides a way to execute custom code whenever the value of a property is set, and the new value differs from the old one. Here’s how you use it:
```swift var myProperty: Int = 0 { didSet { // This code block is executed when 'myProperty' is set, and its value changes print("New value: \(myProperty)") } } ```
In this example, whenever the `myProperty` is modified, the `didSet` property observer is triggered. It gives you access to both the old value (the value before the change) and the new value (the value after the change) of the property. You can use this observer for a variety of purposes, such as logging, updating UI elements, or enforcing certain constraints when a property’s value changes.
Here are some key points to remember about `didSet` property observers:
- Order of Execution: The `didSet` observer is called after the new value has been assigned to the property. This means that you can access the old value, but if you modify the property within `didSet`, it won’t affect the current assignment.
- No Access to Property Name: Unlike the `willSet` observer, the `didSet` observer does not provide an automatic name for the new value. You use the property name itself to refer to the new value within the observer block.
- Multiple Observers: You can have multiple `didSet` observers for a single property, and they will be executed in the order they are declared.
- Conditional Observing: You can conditionally execute code within `didSet` based on certain conditions. This allows you to control when the observer code is executed.
Overall, `didSet` property observers are a valuable tool in Swift for monitoring and responding to changes in property values, helping you add custom behavior to your code when specific properties are modified.
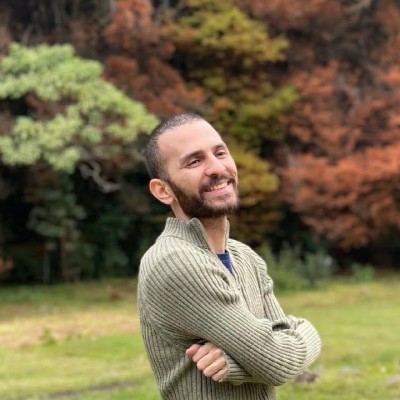
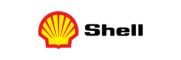