Mastering Error Handling in Swift: Strategies for Robust iOS Apps
Error handling is a critical aspect of iOS app development. When building robust applications, it’s essential to handle errors effectively to ensure a smooth user experience. Swift, the programming language for iOS development, provides powerful mechanisms for managing errors and recovering from unexpected situations. In this blog post, we will delve into strategies and techniques for mastering error handling in Swift, equipping you with the knowledge to build resilient iOS apps.
Understanding Error Handling in Swift
1. What are Errors in Swift?
In Swift, errors are represented by types that conform to the Error protocol. Errors can occur due to various reasons, such as invalid input, resource unavailability, or network failures. By defining custom error types, you can categorize and handle specific types of errors in your app.
2. Throwing and Catching Errors:
Swift uses the throw keyword to throw errors from a function, method, or initializer. On the receiving end, errors can be caught and handled using the do-catch statement. This powerful mechanism allows you to gracefully handle exceptional scenarios.
3. Error Types in Swift:
In addition to the built-in error types provided by Swift, you can define your own custom error types by creating an enum that conforms to the Error protocol. This allows you to create a domain-specific error hierarchy for better error categorization and handling.
Handling Errors with Do-Catch
1. The Do-Catch Statement:
The do-catch statement is the primary construct for handling errors in Swift. It consists of a do block where code that can potentially throw an error is executed, followed by one or more catch blocks that handle specific error cases.
swift do { // Code that can throw errors } catch { // Handle the error }
2. Catching Specific Errors:
You can catch specific types of errors by specifying the error type after the catch keyword. This allows you to handle different error cases differently.
swift do { // Code that can throw errors } catch MyError.invalidInput { // Handle invalid input error } catch MyError.networkFailure { // Handle network failure error } catch { // Handle other types of errors }
3. Rethrowing Errors:
In some cases, you may want to propagate an error to the caller without handling it. By marking a function with the throws keyword, you can rethrow any error that is caught within the function.
swift func performTask() throws { do { // Code that can throw errors } catch { throw error } }
Propagating Errors
1. Throwing Functions:
You can define functions that explicitly declare that they can throw errors using the throws keyword. This allows you to indicate to the caller that the function might fail and propagate any errors that occur.
swift func fetchData() throws -> Data { // Code that can throw errors }
2. Handling Errors in Calling Code:
When calling a throwing function, you have to use one of the error handling techniques. You can use the do-catch statement to handle the error or use the try? or try! keywords to convert the error to an optional or force-unwrap the result, respectively.
swift do { let data = try fetchData() // Handle successful data retrieval } catch { // Handle the error }
3. Using Try? and Try!:
The try? keyword converts an error to an optional value. If the function throws an error, the result will be nil. Conversely, the try! keyword is used when you are confident that the function will not throw an error. If an error does occur, it will result in a runtime error.
swift let data = try? fetchData() // Returns optional Data let data = try! fetchData() // Force-unwraps the result (potential runtime crash)
Custom Error Types
1. Creating Custom Error Enums:
To define custom error types, you can create an enum that conforms to the Error protocol. Each case in the enum represents a specific error scenario, providing clear categorization for error handling.
swift enum NetworkError: Error { case connectionLost case requestTimeout case authenticationFailed }
2. Adding Associated Values to Errors:
You can add associated values to error cases, allowing you to provide additional information about the error. This can be useful for passing contextual details to the error handler.
swift enum FileError: Error { case fileNotFound(path: String) case permissionDenied(user: String) }
3. Error Wrapping and Unwrapping:
When working with libraries or APIs that use a different error system, you may need to wrap or unwrap errors to conform to your app’s error types. This can be achieved using techniques like error bridging or custom error mapping.
Dealing with Asynchronous Code
1. Error Handling with Completion Handlers:
When working with asynchronous operations that use completion handlers, it’s crucial to handle errors appropriately. You can define the completion handler with an optional error parameter, allowing the caller to handle any error that occurs during the operation.
swift func fetchData(completion: (Data?, Error?) -> Void) { // Asynchronous code that can throw errors }
2. Result Type for Asynchronous Operations:
Starting from Swift 5, you can leverage the Result type to represent the success or failure of an operation. This can be particularly useful when dealing with asynchronous code that needs to propagate errors.
swift func fetchData(completion: (Result<Data, Error>) -> Void) { // Asynchronous code that can throw errors }
3. Combining Result Types and Error Handling:
By combining the Result type with error handling techniques, you can build more robust and expressive asynchronous APIs. The Result type allows you to handle success and failure cases separately, making error handling more explicit and readable.
Recovering from Errors:
1. Deferring Work with Defer:
The defer statement allows you to specify a block of code that will be executed regardless of whether an error was thrown or not. This is useful for deferring resource cleanup or performing necessary cleanup actions before exiting a scope.
swift func processFile() throws { let file = openFile() defer { closeFile(file) } // Code that can throw errors }
2. Clean-up Actions with Defer:
Defer statements are executed in the reverse order of their appearance in the code. This ensures that clean-up actions are performed in the expected order, even if multiple defer statements are present.
3. Recovering from Errors with Optional Catch Binding:
In the catch block, you can use optional catch binding to conditionally recover from an error. This allows you to handle an error and continue executing code if a certain condition is met.
swift do { // Code that can throw errors } catch let error as MyError where condition { // Handle the error and continue }
Conclusion
Mastering error handling is crucial for building robust iOS apps. Swift provides powerful mechanisms for managing errors, allowing you to gracefully handle exceptional scenarios and ensure a smooth user experience. By understanding the various error handling strategies, techniques, and code samples discussed in this blog post, you can confidently build resilient iOS applications that handle errors effectively.
Table of Contents
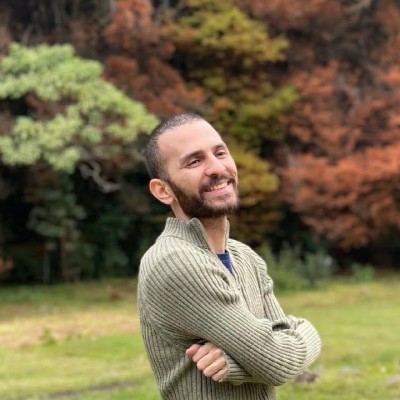
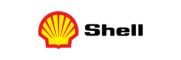