How do I use the ‘@escaping’ attribute in Swift?
In Swift, the ‘@escaping’ attribute is used to annotate a closure parameter within a function or method declaration. This attribute indicates that the closure parameter can potentially outlive the scope of the function in which it is defined. Understanding when and how to use ‘@escaping’ is crucial for handling closures in certain asynchronous scenarios.
Here’s a breakdown of how to use the ‘@escaping’ attribute:
- Non-Escaping Closures (Default Behavior):
By default, closure parameters in Swift functions are assumed to be non-escaping. This means that the closure is executed within the function’s scope and cannot be stored or used outside of that scope. This is the safer option and ensures that the closure doesn’t create strong reference cycles.
```swift func doSomething(completion: () -> Void) { // The closure is non-escaping by default completion() } ```
- Escaping Closures:
When you mark a closure parameter with ‘@escaping’, you’re indicating that the closure can be stored and called later, even after the function has returned. This is often used in asynchronous scenarios where you want to retain the closure for execution at a later time, such as with callbacks or delegates.
```swift func fetchData(completion: @escaping (Result<Data, Error>) -> Void) { // Marking the closure as @escaping because it will be called asynchronously DispatchQueue.global().async { // Simulate fetching data if let data = self.fetchDataFromNetwork() { completion(.success(data)) } else { completion(.failure(NetworkError.fetchFailed)) } } } ```
In the example above, the ‘fetchData’ function uses ‘@escaping’ to indicate that the ‘completion’ closure will be called asynchronously when the data retrieval is complete. This allows the closure to escape the scope of the ‘fetchData’ function and be executed later when the data is available.
Using ‘@escaping’ is essential for handling scenarios where closures need to be retained beyond the lifespan of the enclosing function. However, it also requires careful memory management to avoid strong reference cycles, often achieved by using capture lists or weak references within the closure.
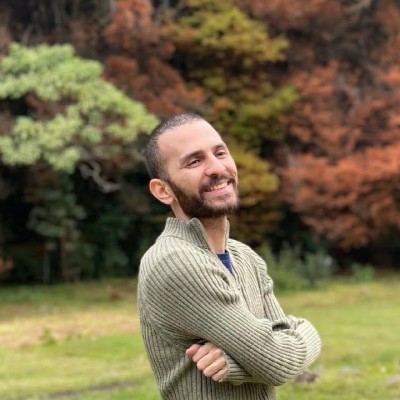
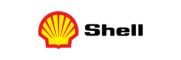