What is a ‘failable initializer’ in Swift?
In Swift, a failable initializer is a special type of initializer that can return `nil` to indicate that an instance of a class, struct, or enum couldn’t be properly initialized due to some validation or initialization condition not being met. Failable initializers are particularly useful when you want to ensure that an object is only created when certain conditions are satisfied, and if those conditions aren’t met, you can gracefully handle the failure by returning `nil`.
Here’s how you define and use failable initializers in Swift:
- Defining a Failable Initializer:
To create a failable initializer, you add a `?` after the `init` keyword and use conditional logic to determine whether the initialization can proceed successfully or not. For example:
```swift struct Temperature { let value: Double init?(_ value: Double) { guard value >= -273.15 else { return nil // Temperature below absolute zero is invalid } self.value = value } } ```
In this example, the `Temperature` struct has a failable initializer that checks if the provided value is valid for representing a temperature. If the value is below absolute zero, the initializer returns `nil`.
- Using a Failable Initializer:
When you use a failable initializer, you wrap the initialization in an optional (`Optional<Type>` or shorthand `Type?`) because it may return `nil`. For instance:
```swift if let freezingPoint = Temperature(0) { print("Water freezes at \(freezingPoint.value)°C.") } else { print("Invalid temperature.") } ```
In this code, if the failable initializer succeeds, you can work with the initialized instance; otherwise, you handle the failure gracefully.
Failable initializers are beneficial for enforcing constraints during object creation, ensuring that instances are only created when certain conditions are met. They provide a clean and safe way to handle potential initialization failures, improving the overall robustness of your Swift code.
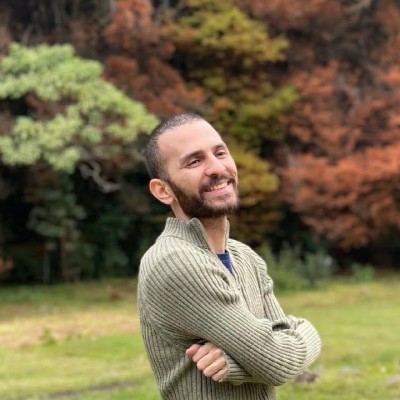
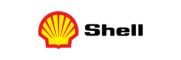