How do I use ‘for-in’ loops in Swift?
Using ‘for-in’ loops in Swift is a fundamental and efficient way to iterate over collections like arrays, dictionaries, sets, and ranges, as well as to perform a set number of iterations. ‘for-in’ loops are concise and offer improved readability compared to traditional C-style ‘for’ loops. Here’s a comprehensive guide on how to use ‘for-in’ loops effectively in Swift:
- Iterating Over Arrays and Other Sequences:
You can use ‘for-in’ loops to iterate over elements in an array or any other sequence type. The loop variable takes on each element of the sequence in each iteration.
```swift let numbers = [1, 2, 3, 4, 5] for number in numbers { print(number) } ```
- Iterating Over Dictionaries:
When iterating over dictionaries, ‘for-in’ loops provide access to both keys and values using a tuple. This allows you to work with both the keys and their corresponding values.
```swift let fruitPrices = ["apple": 1.0, "banana": 0.5, "orange": 0.75] for (fruit, price) in fruitPrices { print("\(fruit) costs $\(price)") } ```
- Iterating with Ranges:
‘for-in’ loops can also be used with ranges to perform a specific number of iterations. This is handy when you need to repeat an action a certain number of times.
```swift for index in 1...5 { print("Iteration \(index)") } ```
- Iterating with Strides:
Swift allows you to iterate over ranges with a specific step using the ‘stride’ function. This is useful for incrementing or decrementing values in controlled steps.
```swift for step in stride(from: 0, to: 10, by: 2) { print(step) } ```
- Enumerating Elements:
If you need both the index and the value of elements while iterating over an array, you can use the ‘enumerated()’ method.
```swift let words = ["apple", "banana", "cherry"] for (index, word) in words.enumerated() { print("Word \(index + 1): \(word)") } ```
‘for-in’ loops provide a clean and concise way to iterate through various types of collections and ranges in Swift. They are a fundamental tool for performing repetitive tasks, processing data, and applying operations to elements within your code.
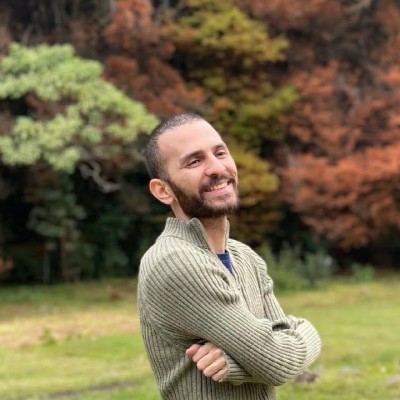
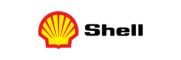