How do I define functions in Swift?
In Swift, a function is a self-contained block of code that performs a specific task or returns a value. Functions are a fundamental building block of Swift applications and play a crucial role in structuring and organizing your code. Here’s how you can define functions effectively in Swift:
Function Declaration:
To define a function in Swift, you use the `func` keyword, followed by the function name and a set of parentheses that can contain optional input parameters. If the function returns a value, you specify the return type using an arrow `->` followed by the data type. Here’s the basic structure:
```swift func functionName(parameter1: Type, parameter2: Type) -> ReturnType { // Function implementation // Use parameters and perform tasks return result // If the function returns a value } ```
Function Parameters:
Functions can take zero or more parameters, and each parameter consists of a name and a data type. Parameters allow you to pass values into the function for processing. For example:
```swift func greet(name: String) { print("Hello, \(name)!") } ```
Return Values:
Functions can return values using the `return` keyword followed by the value or expression to be returned. The return type is specified after the `->` arrow. If a function doesn’t return a value, you use `Void` or simply omit the return type, and Swift infers it as `Void`. For example:
```swift func add(a: Int, b: Int) -> Int { return a + b } ```
Calling Functions:
To use a function, you call it by its name and provide the required arguments. If a function returns a value, you can capture and use it. For example:
```swift let result = add(a: 5, b: 3) // result is 8 greet(name: "Alice") // Prints "Hello, Alice!" ```
External and Internal Parameter Names:
Swift allows you to specify external and internal parameter names for functions. External parameter names are used when calling the function, while internal parameter names are used inside the function. You can use the `externalName internalName` syntax to define them. For example:
```swift func calculateArea(ofRect width: Double, andHeight height: Double) -> Double { return width * height } ```
In this function, `ofRect` and `andHeight` are external parameter names, while `width` and `height` are internal.
In summary, functions in Swift are essential for defining reusable blocks of code that perform specific tasks or return values. You can specify input parameters, return types, and use external and internal parameter names to make your functions expressive and clear. Functions help encapsulate functionality, promote code reusability, and make your code more organized and maintainable.
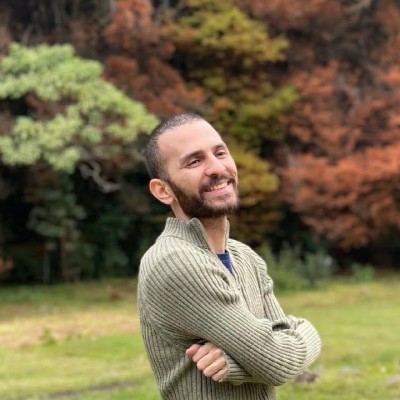
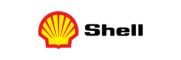