Swift Generics: Harnessing the Power of Type Safety in iOS Development
Introduction to Swift Generics
Swift Generics allow you to write flexible, reusable functions and types that can work with any type, subject to requirements that you define. This powerful feature ensures type safety without sacrificing flexibility, making your code more robust and reducing duplication. Generics are an essential tool in a Swift developer’s toolkit, enabling the creation of components that can adapt to different types while maintaining a strong type system.
Why Use Generics in Swift?
Generics enable you to write code that is both type-safe and reusable. By abstracting types, you can write functions, classes, and structures that work seamlessly with any type, ensuring that your code is both versatile and maintainable. Here’s why generics are so powerful:
- Type Safety: Generics ensure that the types used in your code are consistent and correct at compile time.
- Reusability: Write a function or type once, and use it with any compatible type.
- Flexibility: Generics provide a way to build flexible and adaptable code structures.
Creating Generic Functions
A generic function can work with any type. Here’s how you can define a simple generic function in Swift.
Example: A Generic Function to Swap Values
```swift func swapValues<T>(a: inout T, b: inout T) { let temp = a a = b b = temp } var x = 5 var y = 10 swapValues(a: &x, b: &y) print("x: \(x), y: \(y)") // Output: x: 10, y: 5 ```
In this example, the `swapValues` function can swap the values of any two variables, regardless of their type, as long as they are of the same type.
Using Generic Types in Collections
Swift’s `Array`, `Dictionary`, and `Set` types are all generic collections. You can create your own generic types that provide similar flexibility.
Example: A Generic Stack Structure
```swift struct Stack<Element> { private var elements: [Element] = [] mutating func push(_ element: Element) { elements.append(element) } mutating func pop() -> Element? { return elements.popLast() } func peek() -> Element? { return elements.last } } var intStack = Stack<Int>() intStack.push(1) intStack.push(2) print(intStack.pop()) // Output: Optional(2) var stringStack = Stack<String>() stringStack.push("Hello") stringStack.push("World") print(stringStack.pop()) // Output: Optional("World") ```
This `Stack` structure is generic, meaning it can hold any type of elements, providing a versatile and reusable component in your codebase.
Extending Generics with Constraints
You can impose constraints on generics to ensure that the types used conform to certain protocols or inherit from specific classes.
Example: A Generic Function with a Constraint
```swift func findIndex<T: Equatable>(of valueToFind: T, in array: [T]) -> Int? { for (index, value) in array.enumerated() { if value == valueToFind { return index } } return nil } let numbers = [1, 2, 3, 4, 5] if let index = findIndex(of: 3, in: numbers) { print("Index: \(index)") // Output: Index: 2 } let strings = ["apple", "banana", "cherry"] if let index = findIndex(of: "banana", in: strings) { print("Index: \(index)") // Output: Index: 1 } ```
Here, the `findIndex` function works with any type that conforms to the `Equatable` protocol, ensuring that the elements can be compared for equality.
Practical Use Cases of Generics in iOS Development
Generics are not just a theoretical concept; they have practical applications in real-world iOS development. Some common use cases include:
– Reusable Networking Code: Create generic functions for handling network requests that can return different data types.
– Custom Data Structures: Develop custom data structures, like linked lists or queues, that can handle any data type.
– Model-View-ViewModel (MVVM) Architecture: Use generics to create flexible view models that can adapt to different types of models.
Conclusion
Swift Generics are a powerful feature that enhances both the type safety and reusability of your code. By understanding and utilizing generics effectively, you can write more flexible, maintainable, and robust iOS applications. Whether you’re building data structures, handling network responses, or implementing complex architectures, generics will help you achieve cleaner and more efficient code.
Further Reading:
Table of Contents
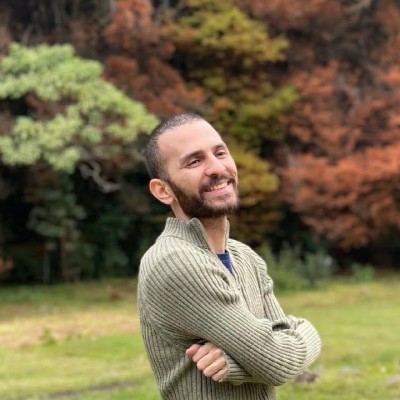
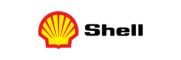