What is the ‘guard case’ statement in Swift?
In Swift, the `guard case` statement is a powerful construct used within a `guard` statement to conditionally and safely unwrap optional values while also checking for a specific condition. It’s particularly useful when you want to ensure that a certain condition is met and that the unwrapped value satisfies specific criteria, all in a concise and readable manner.
Here’s how the `guard case` statement works:
- Defining a Guard Statement: A `guard` statement is used to require that a specific condition is true; otherwise, it exits the current scope, often with an `else` clause that contains code to run when the condition is not met. It’s commonly used for early exit from a function or method to handle invalid cases gracefully.
- Using ‘guard case’: Within a `guard` statement, you can use the `guard case` statement to check whether an optional value matches a particular pattern (e.g., enum case or type) and whether a condition is true simultaneously. If the value doesn’t match the pattern or the condition is false, the `else` block is executed, typically containing code to handle the failure case.
Here’s a simplified example:
```swift enum TrafficLight { case red, yellow, green } func handleTrafficLight(_ light: TrafficLight?) { guard case .green = light, light != nil else { print("Invalid traffic light state") return } // Code to handle when the traffic light is green print("You can proceed") } // Usage let currentLight: TrafficLight? = .green handleTrafficLight(currentLight) // Outputs: "You can proceed" ```
In this example, the `guard case .green = light, light != nil` statement ensures that `light` is both not `nil` and matches the `.green` pattern. If either condition fails, the `else` block is executed.
The `guard case` statement is a concise way to safely unwrap and validate values, making your code more robust by handling potentially problematic cases early and clearly expressing your intent in terms of pattern matching and conditions. It’s commonly used in Swift to improve code readability and safety.
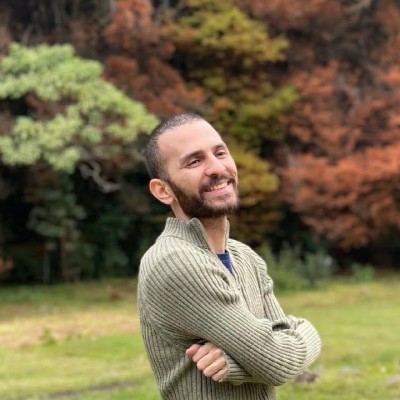
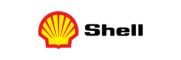