What is a guard statement in Swift?
In Swift, a guard statement is a control flow structure used to ensure that a specific condition is met in order to proceed with the execution of code. It is primarily used for early exits from a block of code if certain conditions are not satisfied, making your code more readable and helping to avoid deeply nested if-else statements.
Here’s the basic structure of a guard statement:
```swift guard condition else { // Code to execute if the condition is not met // Typically, this includes error handling, cleanup, or early return } ```
Key points about the guard statement:
- Condition: The guard statement starts with a condition that evaluates to a Boolean value. If the condition is true, execution continues normally. If it’s false, the code inside the `else` block is executed.
- Early Exit: The primary purpose of guard is to facilitate early exit from a function, method, or code block if a required condition is not met. This is particularly useful for validating inputs, unwrapping optionals, or checking preconditions.
- Error Handling: One common use of guard is for error handling. You can use it to check whether certain conditions for a function or method are satisfied. If not, you can return an error or handle it appropriately within the `else` block.
- Optional Unwrapping: Guard is also often used to safely unwrap optionals. If an optional contains a value, it proceeds with execution; otherwise, it safely exits, preventing nil-related runtime crashes.
Here’s an example of guard statement in action:
```swift func calculateSquareRoot(_ value: Double?) -> Double? { guard let validValue = value, validValue >= 0 else { return nil // Return nil if value is nil or negative } return sqrt(validValue) // Calculate and return square root } ```
In this example, the guard statement ensures that the input value is not nil and is non-negative before attempting to calculate its square root. If the condition is not met, it exits early and returns nil, indicating an invalid input.
In summary, the guard statement in Swift is a powerful tool for improving code readability and ensuring that necessary conditions are met before proceeding with execution. It’s commonly used for error handling, optional unwrapping, and validating inputs, helping developers write more robust and maintainable code.
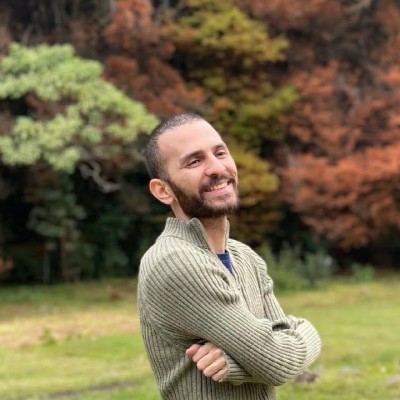
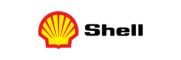