How do I handle errors in Swift?
Handling errors in Swift is an essential part of writing robust and reliable code. Swift provides a comprehensive error-handling mechanism that allows you to gracefully manage unexpected situations and failures. The primary constructs for error handling in Swift are the `try`, `catch`, and `throw` keywords, along with error types and protocols.
Here’s how you handle errors in Swift:
- Error Types: In Swift, errors are represented as values that conform to the `Error` protocol or its subtypes. You can use predefined error types like `NSError`, or create custom error types by defining your own structs or enums that conform to the `Error` protocol.
- Throwing Errors: To signal an error in your code, use the `throw` keyword followed by an error value. This is typically done within a function or method when an exceptional condition occurs.
- Error Propagation: When a function can throw an error, you mark it with the `throws` keyword in its declaration. This indicates that the function might encounter an error during its execution and allows callers to handle the error.
- Handling Errors: To catch and handle errors, use the `do-catch` statement. Code that might throw errors is enclosed in a `do` block, and any errors are caught and handled in one or more `catch` blocks. You can have multiple `catch` blocks to handle different types of errors or provide different error-handling logic.
- Defer Statements: Swift also supports `defer` statements, which allow you to specify code that will be executed regardless of whether an error was thrown or not. This is useful for cleanup operations.
Here’s a simplified example of error handling in Swift:
```swift enum FileError: Error { case notFound case permissionDenied } func openFile() throws { let fileExists = /* check if file exists */ if !fileExists { throw FileError.notFound } let hasPermission = /* check file permissions */ if !hasPermission { throw FileError.permissionDenied } // File is open successfully } do { try openFile() // Continue with file operations } catch FileError.notFound { print("File not found.") } catch FileError.permissionDenied { print("Permission denied.") } catch { print("An unexpected error occurred: \(error)") } ```
In this example, the `openFile` function can throw errors of type `FileError`. We use a `do-catch` block to call `openFile()` and handle different error cases. If any error is thrown, the appropriate `catch` block is executed.
Overall, Swift’s error-handling mechanism ensures that your code can gracefully recover from errors, report meaningful error messages, and maintain code reliability even in the face of unexpected issues.
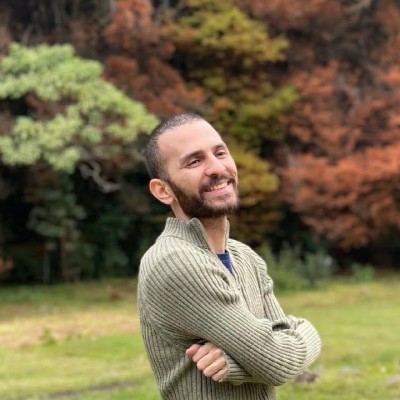
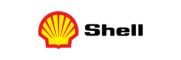