Intuitive Health Data Management: Swift and HealthKit Explained with Code Examples
The modern world is filled with an abundance of digital tools to help users monitor their health and fitness journey. Apple’s HealthKit is at the forefront, providing developers with an efficient way to integrate health and fitness data into their apps. If you’re building an iOS application using Swift and want to tap into this vast ecosystem, you’re in the right place. Whether you’re looking to hire Swift developers or take the DIY route, this article will provide an overview of how to seamlessly integrate HealthKit into your Swift apps with practical examples.
Table of Contents
1. What is HealthKit?
HealthKit is a framework introduced by Apple to consolidate health and fitness data from various apps and devices into one cohesive health profile on a user’s device. With user permission, your app can read from or write to this database, creating a centralized place for all health-related data.
1.1 Setting Up HealthKit
- Add HealthKit to your project.
– Go to your project settings.
– Under the `Capabilities` tab, turn on HealthKit.
- Check if HealthKit is available on the device.
Not all devices support HealthKit, and some features are only available on specific devices.
```swift if HKHealthStore.isHealthDataAvailable() { // HealthKit is available } ```
1.2 Fetching and Saving Data with HealthKit
Types of Health Data*: HealthKit categorizes data into:
- Samples: e.g., blood pressure, heart rate.
- Characteristics: e.g., date of birth, blood type.
Here are some practical examples:
- Requesting Permission
Before accessing a user’s health data, you need to request permission. Specify the types of data you wish to access.
```swift let healthStore = HKHealthStore() let readDataTypes: Set = [ HKObjectType.quantityType(forIdentifier: .heartRate)!, HKObjectType.quantityType(forIdentifier: .activeEnergyBurned)! ] let writeDataTypes: Set = [ HKObjectType.quantityType(forIdentifier: .dietaryEnergyConsumed)! ] healthStore.requestAuthorization(toShare: writeDataTypes, read: readDataTypes) { (success, error) in if !success { // Handle error } } ```
- Reading Data
Fetch the latest heart rate for a user:
```swift let heartRateType = HKQuantityType.quantityType(forIdentifier: .heartRate)! let sortDescriptor = NSSortDescriptor(key: HKSampleSortIdentifierStartDate, ascending: false) let query = HKSampleQuery(sampleType: heartRateType, predicate: nil, limit: 1, sortDescriptors: [sortDescriptor]) { (query, results, error) in guard let samples = results as? [HKQuantitySample], let latestSample = samples.first else { return } let heartRate = latestSample.quantity.doubleValue(for: HKUnit.count().unitDivided(by: HKUnit.minute())) print("Latest heart rate: \(heartRate) bpm") } healthStore.execute(query) ```
- Writing Data
Log a dietary energy consumption:
```swift let energyQuantity = HKQuantity(unit: HKUnit.kilocalorie(), doubleValue: 500) let energyType = HKQuantityType.quantityType(forIdentifier: .dietaryEnergyConsumed)! let energySample = HKQuantitySample(type: energyType, quantity: energyQuantity, start: Date(), end: Date()) healthStore.save(energySample) { (success, error) in if !success { // Handle error } } ```
2. Integrating HealthKit with Workouts
Workouts are a special type of data in HealthKit. You can track and save a wide range of workouts, from running and cycling to yoga.
2.1 Starting a Workout Session:
```swift let workoutConfig = HKWorkoutConfiguration() workoutConfig.activityType = .running workoutConfig.locationType = .outdoor let workoutSession = try! HKWorkoutSession(healthStore: healthStore, configuration: workoutConfig) workoutSession.startActivity(with: Date()) ```
2.2 Ending a Workout Session:
```swift workoutSession.end() let workout = HKWorkout(activityType: .running, start: workoutSession.startDate, end: Date(), workoutEvents: nil, totalEnergyBurned: nil, totalDistance: nil, device: nil, metadata: nil) healthStore.save(workout, withCompletion: { (success, error) in if !success { // Handle error } }) ```
Conclusion
Swift, when combined with HealthKit, becomes a potent tool for iOS developers who are aiming to create comprehensive health and fitness applications. For those looking to scale or expedite their projects, they often opt to hire Swift developers. The examples provided above give just a glimpse into the wide range of functionalities offered by HealthKit. The key is to always respect the user’s privacy, ask for explicit permissions, and leverage the data to offer enhanced value. As health and fitness continue to gain prominence in the digital age, the potential for innovative apps in this sector is vast and untapped. Whether you’re coding on your own or planning to hire Swift developers for specialized input, the future looks promising. Happy coding!
Table of Contents
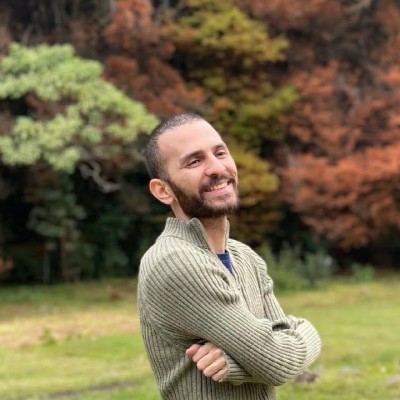
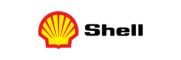