How do I use inheritance in Swift?
Inheritance is a fundamental concept in object-oriented programming (OOP) that allows you to create new classes (known as subclasses or derived classes) based on existing classes (known as base classes or superclasses). In Swift, you can use inheritance to model relationships between classes, promote code reuse, and create a hierarchy of classes with shared behaviors and properties.
Here’s how to use inheritance effectively in Swift:
- Define a Base Class: Start by creating a base class, which contains the common properties and methods shared among the derived classes. This class serves as the foundation for the inheritance hierarchy.
- Create Subclasses: To create subclasses, use the `class` keyword followed by the subclass name, a colon, and the name of the base class. Subclasses inherit all properties and methods from the base class and can add their own properties and methods or override existing ones.
- Access Superclass Members: In a subclass, you can access properties and methods from the base class using the `super` keyword. This allows you to call the superclass’s implementations or modify their behavior in the subclass.
- Initialization: When initializing a subclass, you should call the designated initializer of the superclass using `super.init()`. This ensures that the superclass’s properties are properly initialized before adding any subclass-specific setup.
- Method Overriding: Subclasses can override methods and computed properties from the base class by using the `override` keyword. This allows you to provide custom implementations while preserving the same interface.
- Inheritance Hierarchy: You can create multiple levels of inheritance by having subclasses that become base classes for further subclasses, creating a hierarchy of related classes.
Here’s a simplified example in Swift:
```swift class Animal { var name: String init(name: String) { self.name = name } func makeSound() { print("Animal makes a sound") } } class Dog: Animal { override func makeSound() { print("Dog barks") } func fetch() { print("Dog fetches a ball") } } ```
In this example, we have a base class `Animal` and a subclass `Dog`. The `Dog` class inherits the `name` property and the `makeSound()` method from `Animal` and adds its own method `fetch()`. It also overrides the `makeSound()` method to provide a custom implementation.
In summary, inheritance in Swift is a powerful mechanism for creating hierarchical relationships between classes, allowing you to share and extend functionality in an organized and efficient way. It promotes code reuse, simplifies maintenance, and helps you model real-world relationships in your software design.
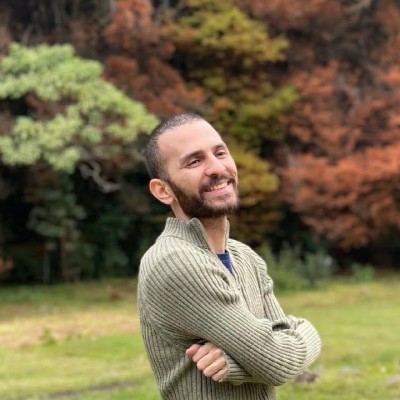
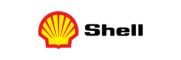