Swift Internationalization: Making iOS Apps Multilingual
Internationalization (i18n) is the process of designing an app to support multiple languages and regions. Swift, Apple’s powerful programming language for iOS development, offers robust tools and frameworks to help developers internationalize their applications effectively. This blog explores how Swift can be used to make iOS apps multilingual and provides practical examples for implementing internationalization.
Understanding Internationalization
Internationalization involves preparing your app to support different languages and regional formats. It encompasses various aspects, including translating user interface elements, formatting dates and numbers according to regional preferences, and adapting layouts for different languages. Effective internationalization ensures that your app provides a consistent and user-friendly experience to users across different languages and regions.
Using Swift for Internationalization
Swift provides several features and frameworks that simplify the process of internationalizing iOS apps. Here are key aspects and code examples demonstrating how to use Swift for internationalization.
1. Localizing Strings and Resources
The first step in making an iOS app multilingual is to localize its strings and resources. Swift uses the `Localizable.strings` file to store translations of text used in the app.
Example: Localizing Strings
1. Create a `Localizable.strings` file for each language you want to support. For example, you might have `Localizable.strings` files for English (`en.lproj`) and Spanish (`es.lproj`).
2. Add translations to each file:
English (`en.lproj/Localizable.strings`): ```plaintext "welcome_message" = "Welcome to our app!"; ``` Spanish (`es.lproj/Localizable.strings`): ```plaintext "welcome_message" = "¡Bienvenido a nuestra aplicación!"; ```
3. Use the `NSLocalizedString` function in your Swift code to fetch the localized string:
```swift let welcomeMessage = NSLocalizedString("welcome_message", comment: "Welcome message for the app") print(welcomeMessage) ```
2. Formatting Dates and Numbers
Different regions use different formats for dates, numbers, and currencies. Swift’s `NumberFormatter` and `DateFormatter` classes help ensure that these are formatted correctly based on the user’s locale.
Example: Formatting Dates
```swift import Foundation let dateFormatter = DateFormatter() dateFormatter.dateStyle = .long dateFormatter.locale = Locale(identifier: "es_ES") // Spanish locale let date = Date() let formattedDate = dateFormatter.string(from: date) print("Formatted date: \(formattedDate)") ```
Example: Formatting Numbers
```swift import Foundation let numberFormatter = NumberFormatter() numberFormatter.numberStyle = .currency numberFormatter.locale = Locale(identifier: "fr_FR") // French locale let amount = 1234.56 let formattedAmount = numberFormatter.string(from: NSNumber(value: amount)) print("Formatted amount: \(formattedAmount ?? "")") ```
3. Adapting Layouts for Different Languages
Languages can vary in length and direction, which might affect the layout of your app. Swift provides tools for creating adaptive layouts using Auto Layout and size classes.
Example: Adaptive Layout with Auto Layout
In Interface Builder, use Auto Layout constraints to ensure that your UI elements adapt to different languages and screen sizes. For right-to-left languages, you might need to adjust layouts to accommodate text direction.
4. Integrating Localization with SwiftUI
SwiftUI offers built-in support for localization. You can use localized strings in SwiftUI views similarly to how you use them in UIKit.
Example: Using Localized Strings in SwiftUI
```swift import SwiftUI struct ContentView: View { var body: some View { Text(NSLocalizedString("welcome_message", comment: "Welcome message for the app")) .padding() } } ```
Conclusion
Swift provides a comprehensive set of tools for internationalizing iOS applications. By localizing strings and resources, formatting dates and numbers, adapting layouts, and integrating with SwiftUI, you can create multilingual apps that offer a seamless experience for users worldwide. Leveraging these capabilities effectively will help you reach a broader audience and enhance your app’s accessibility.
Further Reading:
Table of Contents
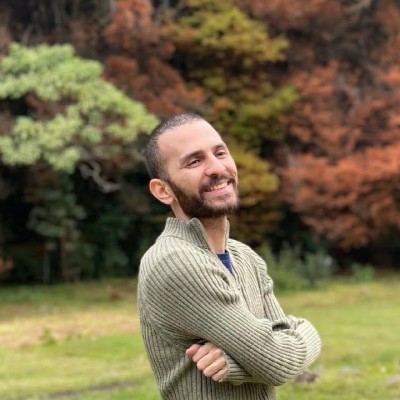
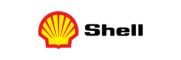