How do I use the ‘is’ operator in Swift?
The ‘is’ operator in Swift is a fundamental tool for type checking and type casting. It allows you to check if an instance is of a certain type or conforms to a specific protocol. This operator is essential when you want to work with objects in a safe and flexible way, especially in scenarios involving inheritance and polymorphism.
Here’s how you can use the ‘is’ operator in Swift:
- Type Checking: The most common use of ‘is’ is for type checking. You can use it to determine if an instance is an instance of a particular class or a subclass. For example:
```swift if myInstance is MyClass { // Perform actions specific to MyClass } ```
This condition will evaluate to true if `myInstance` is an instance of `MyClass` or any of its subclasses.
- Type Casting: The ‘is’ operator is often used in conjunction with type casting operators like ‘as?’ or ‘as!’ to safely downcast an instance to a specific class or protocol. For instance:
```swift if let myObject = someInstance as? MyType { // Successfully casted to MyType, use myObject here } ```
Here, if `someInstance` is of type `MyType` or a subclass, it will be safely casted to `MyType`, and you can work with it as such.
- Protocol Conformance: You can also use ‘is’ to check if an object conforms to a certain protocol:
```swift if myObject is MyProtocol { // myObject conforms to MyProtocol } ```
This check is useful when you want to ensure that an object can be used in a protocol-specific context.
The ‘is’ operator is crucial for ensuring the correctness and safety of your code when dealing with polymorphic and dynamic scenarios. It allows you to make decisions based on the actual types of objects at runtime, enabling you to write more flexible and robust Swift applications.
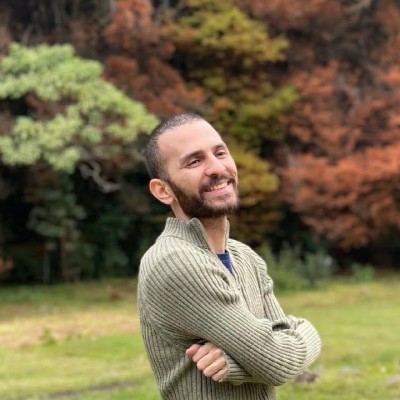
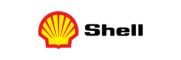