What is the use of ‘lazy’ keyword in Swift?
In Swift, the ‘lazy’ keyword is used to declare a property as “lazy,” which means its initial value is not calculated until the property is first accessed. This feature is particularly useful when you have a property whose value may not be needed immediately, or when the calculation of the initial value is resource-intensive. Here’s how the ‘lazy’ keyword works and its common use cases:
Lazy Initialization: When you mark a property as ‘lazy,’ Swift ensures that the initial value of the property is only computed when it is first accessed. Until that point, the property remains uninitialized, saving computational resources and memory. This is especially beneficial for properties that involve complex calculations or expensive operations.
```swift class Example { lazy var expensiveOperationResult: Int = { // Perform some resource-intensive calculation return 42 }() } ```
Deferred Initialization: ‘lazy’ properties are also suitable for deferring the initialization of a property until it is actually required, which can be crucial for performance optimization. This means that if the property is never accessed during the execution of the program, the expensive calculation is never performed.
Common Use Cases: The ‘lazy’ keyword is commonly used in scenarios where you want to delay the initialization of properties, such as creating singleton instances, loading data from files or networks only when needed, or initializing properties that are used infrequently. It helps improve the efficiency of your code by avoiding unnecessary work upfront.
```swift class FileManager { lazy var documentDirectory: URL = { let urls = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask) return urls[0] }() } ```
In summary, the ‘lazy’ keyword in Swift is a valuable tool for optimizing resource usage and improving the performance of your code. It allows properties to be initialized only when they are accessed, helping you manage the allocation of resources efficiently and avoid unnecessary work during program execution.
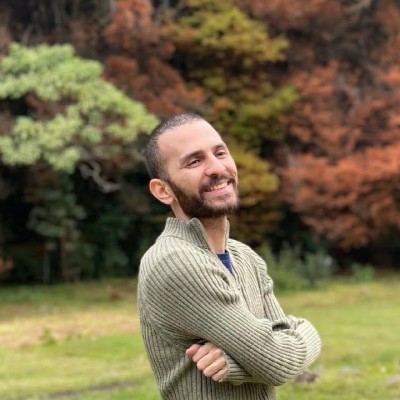
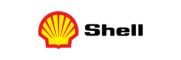