Discover How Swift Libraries Can Skyrocket Your iOS App’s Performance
Developing applications for iOS can often feel like an intricate dance involving creativity, code, and an understanding of users’ needs. To make this dance more elegant and efficient, developers frequently employ Swift libraries, which offer pre-written code for certain functionalities, enabling faster development, code optimization, and enhancement of app features.
Table of Contents
If this process seems daunting, you may opt to hire Swift developers who are experts in integrating these libraries to ensure the seamless functionality of your iOS application.
This blog post will walk you through the process of integrating Swift libraries, a common task for Swift developers, into your iOS application. Additionally, it will provide examples of several useful libraries that can help you enhance your app’s functionality, showcasing the kind of expertise you can expect when you hire Swift developers.
1. The Importance of Swift Libraries
Swift libraries essentially provide prepackaged sets of functionality that can be imported into your app. This prevents you from having to write all your code from scratch, thereby allowing you to focus more on the unique aspects of your app. Not only does this save time and effort, but it also increases the reliability of your app, as these libraries are often extensively tested and widely used, minimizing the likelihood of bugs and issues.
2. How to Integrate Swift Libraries into your iOS App
There are several ways to integrate Swift libraries into your project, but the two most common methods are through the Swift Package Manager (SPM) and CocoaPods.
2.1. Swift Package Manager (SPM)
Swift Package Manager is a tool that comes integrated with Swift, which allows you to manage external libraries in your project. Here is a basic guide on how to use it:
- Open your project in Xcode.
- Click on `File -> Swift Packages -> Add Package Dependency…`
- In the search box, input the URL of the repository containing the Swift package you wish to include.
- Select the version range you want to include.
- Click “Next”, then “Finish”.
Your Swift Package is now integrated into your project!
2.2. CocoaPods
CocoaPods is another tool used for dependency management in Swift and Objective-C projects. To use CocoaPods, follow these steps:
- Install CocoaPods by opening Terminal and running the command `sudo gem install cocoapods`.
- Go to your Xcode project directory in Terminal using the `cd` command.
- Run the command `pod init` to create a Podfile.
- Open the Podfile using `open -a Xcode Podfile`.
- Under the target section, add the library you want to use, like so: `pod ‘LibraryName’`.
- Save and close the Podfile.
- Go back to Terminal and run `pod install`.
That’s it! Your library is now integrated into your Xcode project.
3. 5 Swift Libraries That Can Enhance Your iOS App
Now that you know how to integrate Swift libraries into your iOS app let’s discuss some popular libraries that can significantly improve your app’s functionality.
3.1. Alamofire
Alamofire is a popular library for HTTP networking in Swift. It simplifies a range of common networking tasks, including sending GET, POST, and other types of HTTP requests, downloading files, and much more.
Here is an example of how you can send a GET request using Alamofire:
```swift Alamofire.request("https://api.github.com/users/octocat").responseJSON { response in if let json = response.result.value { print("JSON: \(json)") } } ```
3.2. Kingfisher
Kingfisher is a powerful, open-source library used for downloading and caching images from the web. It provides a simple and straightforward way to handle remote image downloads while also offering customizable caching, image processing, and image viewing.
Here is how you can use Kingfisher to download and display an image in an ImageView:
```swift let url = URL(string: "https://example.com/image.jpg") imageView.kf.setImage(with: url) ```
3.3. RealmSwift
RealmSwift is an alternative to CoreData and SQLite for data persistence in iOS apps. It’s simple to use and provides powerful functionalities, such as real-time automatic data synchronization, data encryption, and querying.
Here is a simple example of how to define a model and store data using RealmSwift:
```swift // Define your model class Dog: Object { @Persisted var name: String @Persisted var age: Int } // Use it let myDog = Dog() myDog.name = "Rex" myDog.age = 1 let realm = try! Realm() try! realm.write { realm.add(myDog) } ```
3.4. SwiftyJSON
SwiftyJSON makes it easy to handle JSON data in Swift. It drastically simplifies parsing JSON and handling potential parsing errors.
Here’s an example of how to use SwiftyJSON to parse JSON data:
```swift let json = JSON(parseJSON: jsonString) let name = json["name"].stringValue ```
3.5. SnapKit
SnapKit is a DSL that allows you to create iOS and OS X Auto Layout constraints with ease and less code. It simplifies the process of setting up constraints programmatically and improves code readability.
Here’s a simple example of SnapKit usage:
```swift box.snp.makeConstraints { (make) -> Void in make.width.height.equalTo(50) make.center.equalTo(self.view) } ```
Conclusion
Swift libraries can save you a ton of time, effort, and lines of code by providing you with tested, ready-to-use functionalities. When you hire Swift developers, they can effectively integrate libraries like Alamofire, Kingfisher, RealmSwift, SwiftyJSON, and SnapKit into your app, significantly boosting its functionality, and speeding up your development process. This can ultimately result in a more polished, robust, and efficient app.
Remember that the power of libraries lies not only in the functionalities they provide but also in how you or the Swift developers you hire use them to craft your app’s unique functionalities and experiences. Happy coding!
Table of Contents
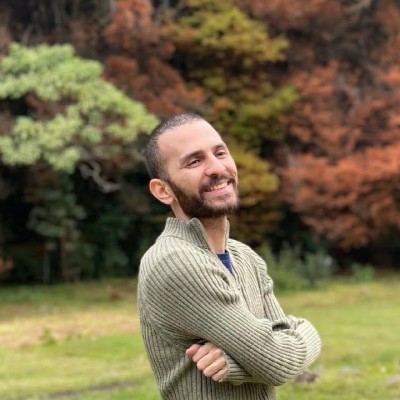
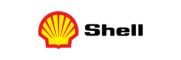