What is the ‘map’ function in Swift?
In Swift, the ‘map’ function is a higher-order function that operates on sequences such as arrays, dictionaries, and other collection types. It allows you to apply a transformation or operation to each element in the sequence and return a new sequence with the modified elements. The ‘map’ function is a fundamental building block for working with collections in a functional and declarative way.
Here’s a detailed explanation of how the ‘map’ function works:
- Transformation: The primary purpose of ‘map’ is to transform each element of a collection into a new element based on a provided transformation function. This transformation can be anything from modifying the element’s value, extracting specific properties, or even performing complex calculations.
- Immutability: ‘map’ doesn’t modify the original collection but creates a new one with the transformed elements. This ensures immutability, a fundamental concept in functional programming. Immutability helps prevent unintended side effects and makes code more predictable.
- Syntax: In Swift, you typically use ‘map’ as a method on an array or another collection. The method takes a closure or a function as an argument, defining the transformation for each element. Here’s a simplified example using an array of integers:
```swift let numbers = [1, 2, 3, 4, 5] let squaredNumbers = numbers.map { $0 * $0 } // squaredNumbers is now [1, 4, 9, 16, 25] ```
In this example, ‘map’ applies the closure `{ $0 * $0 }` to each element in the ‘numbers’ array, squaring each number and returning a new array, ‘squaredNumbers,’ with the transformed values.
- Generic: The ‘map’ function is generic, meaning it can be used with various types of sequences, including arrays, sets, dictionaries, and more. You can also use ‘map’ with custom types by providing a suitable transformation function.
Overall, the ‘map’ function in Swift promotes a clean and expressive way to transform elements within a collection while maintaining the immutability of the original data, making it a valuable tool in functional programming and data manipulation tasks.
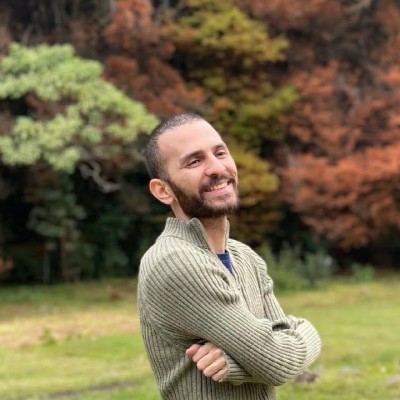
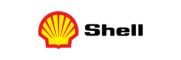