Swift and MapKit: Incorporating Maps and Location Services into iOS Apps
Maps and location-based services are integral to many modern iOS applications, from navigation to location-based recommendations. MapKit, Apple’s framework for integrating maps into iOS apps, offers powerful capabilities for displaying and interacting with maps. This article explores how to use Swift and MapKit to incorporate maps and location services into your iOS apps, providing practical examples and code snippets to get you started.
Understanding MapKit
MapKit is a framework provided by Apple that allows developers to embed maps directly into their iOS applications. It supports various functionalities, including displaying annotations, overlays, and user location. By using MapKit, developers can create interactive and informative map experiences tailored to their app’s needs.
Using Swift and MapKit for Maps and Location Services
Swift, with its modern syntax and powerful features, is well-suited for working with MapKit. Below are some key aspects and code examples demonstrating how to use Swift and MapKit for integrating maps and location services.
1. Setting Up MapKit in Your Project
To start using MapKit, you need to import the framework and configure your project. Ensure that you have the necessary permissions for location services by adding the appropriate keys to your app’s `Info.plist` file.
Example: Basic MapView Setup
```swift import UIKit import MapKit class ViewController: UIViewController { @IBOutlet weak var mapView: MKMapView! override func viewDidLoad() { super.viewDidLoad() // Set initial location let initialLocation = CLLocation(latitude: 37.7749, longitude: -122.4194) // San Francisco let region = MKCoordinateRegion(center: initialLocation.coordinate, latitudinalMeters: 1000, longitudinalMeters: 1000) mapView.setRegion(region, animated: true) } } ```
2. Adding Annotations to the Map
Annotations are used to mark specific locations on the map with custom icons or labels. You can add annotations to represent points of interest or user locations.
Example: Adding a Custom Annotation
```swift import UIKit import MapKit class ViewController: UIViewController, MKMapViewDelegate { @IBOutlet weak var mapView: MKMapView! override func viewDidLoad() { super.viewDidLoad() mapView.delegate = self // Add annotation let annotation = MKPointAnnotation() annotation.title = "San Francisco" annotation.coordinate = CLLocationCoordinate2D(latitude: 37.7749, longitude: -122.4194) mapView.addAnnotation(annotation) } func mapView(_ mapView: MKMapView, viewFor annotation: MKAnnotation) -> MKAnnotationView? { let annotationView = MKPinAnnotationView(annotation: annotation, reuseIdentifier: nil) annotationView.pinTintColor = .red return annotationView } } ```
3. Handling User Location
To provide location-based services, you need to access the user’s location. MapKit integrates seamlessly with Core Location to provide real-time location data.
Example: Tracking User Location
```swift import UIKit import MapKit import CoreLocation class ViewController: UIViewController, CLLocationManagerDelegate { @IBOutlet weak var mapView: MKMapView! let locationManager = CLLocationManager() override func viewDidLoad() { super.viewDidLoad() locationManager.delegate = self locationManager.requestWhenInUseAuthorization() mapView.showsUserLocation = true } func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) { if let location = locations.last { let region = MKCoordinateRegion(center: location.coordinate, latitudinalMeters: 1000, longitudinalMeters: 1000) mapView.setRegion(region, animated: true) } } } ```
4. Adding Overlays for Enhanced Mapping
Overlays allow you to add custom shapes or images on top of the map, such as routes or areas of interest.
Example: Drawing a Circle Overlay
```swift import UIKit import MapKit class ViewController: UIViewController { @IBOutlet weak var mapView: MKMapView! override func viewDidLoad() { super.viewDidLoad() // Add circle overlay let circle = MKCircle(center: CLLocationCoordinate2D(latitude: 37.7749, longitude: -122.4194), radius: 500) mapView.addOverlay(circle) } func mapView(_ mapView: MKMapView, rendererFor overlay: MKOverlay) -> MKOverlayRenderer { if let circle = overlay as? MKCircle { let renderer = MKCircleRenderer(circle: circle) renderer.fillColor = UIColor.red.withAlphaComponent(0.5) renderer.strokeColor = .red renderer.lineWidth = 2 return renderer } return MKOverlayRenderer() } } ```
Conclusion
Swift and MapKit offer a powerful combination for integrating maps and location services into iOS applications. From setting up basic maps and adding annotations to tracking user location and creating overlays, these tools provide a comprehensive suite of features to enhance your app’s user experience. By leveraging these capabilities, you can build engaging and location-aware applications that stand out in the competitive app market.
Further Reading:
Table of Contents
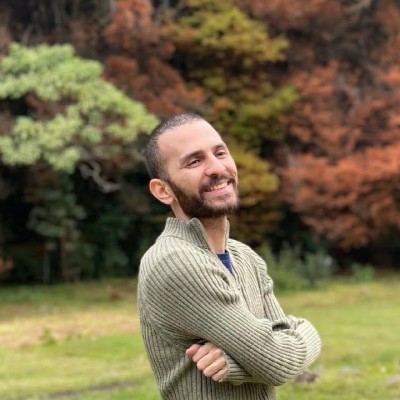
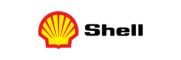