Boost Your iOS App’s Efficiency with Swift Memory Management Best Practices
Swift, the powerful language behind most of the modern iOS, macOS, watchOS, and tvOS apps, provides an efficient and automatic memory management system. However, if not understood well, memory issues such as leaks can cause headaches for developers. To ensure your applications are running optimally, many choose to hire Swift developers for specialized insights. Let’s explore the fundamentals of Swift’s memory management and discuss best practices for optimizing resource usage in iOS apps.
Table of Contents
1. Automatic Reference Counting (ARC)
Swift uses Automatic Reference Counting (ARC) to track and manage the application’s memory usage. Whenever a variable holds an instance of an object, ARC increments the ‘reference count’ of that object. When that reference is no longer needed, ARC decrements the count. When the count drops to zero, the memory occupied by that object is released.
Example:
```swift class Dog { var name: String init(name: String) { self.name = name } deinit { print("\(name) is being deinitialized!") } } var myDog: Dog? = Dog(name: "Rusty") myDog = nil // Prints: Rusty is being deinitialized! ```
In the example above, ARC automatically deinitializes the `Dog` instance when `myDog` is set to nil, indicating that there’s no more reference to the object.
2. Strong Reference Cycles
While ARC handles most of the memory management efficiently, it has its limitations. One of them is the inability to handle reference cycles on its own.
Example:
```swift class Owner { var pet: Dog? } let john = Owner() let rusty = Dog(name: "Rusty") john.pet = rusty ```
In the example above, `Owner` has a strong reference to `Dog` and if `Dog` had a reference back to `Owner`, a strong reference cycle would be created. This can lead to memory leaks.
3. Weak and Unowned References
Swift provides two ways to break reference cycles: `weak` and `unowned` references.
– weak: Used when the reference can become nil at some point.
– unowned: Used when the reference should never be nil once initialized.
Example:
```swift class Owner { var pet: Dog? deinit { print("Owner is being deinitialized!") } } class Dog { weak var owner: Owner? var name: String init(name: String) { self.name = name } deinit { print("\(name) is being deinitialized!") } } var john: Owner? = Owner() var rusty: Dog? = Dog(name: "Rusty") john?.pet = rusty rusty?.owner = john john = nil // Prints: Owner is being deinitialized! rusty = nil // Prints: Rusty is being deinitialized! ```
In this case, `Dog` has a weak reference to `Owner`, thus breaking the reference cycle.
4. Closures and Memory Leaks
Closures can capture and store references to any constants and variables within the context they’re defined. This is known as closing over those references. This can lead to strong reference cycles.
Example:
```swift class TaskManager { var taskCompletion: (() -> Void)? func completeTask() { taskCompletion?() } } let manager = TaskManager() manager.taskCompletion = { manager.completeTask() } ```
Here, `manager`’s closure captures a strong reference to `manager`. To break this cycle, Swift offers capture lists.
```swift manager.taskCompletion = { [weak self] in self?.completeTask() } ```
5. Profiling with Instruments
While coding best practices help, it’s essential to profile your app regularly using Instruments in Xcode. The Leaks and Allocations instruments help identify memory leaks and excess memory allocations.
6. Best Practices for Swift Memory Management
- Understand ARC: Spend time understanding the nuances of ARC and its functioning. Familiarity with its working helps in preventing reference cycles.
- Use `weak` and `unowned` judiciously: Always decide between `weak` and `unowned` based on the lifecycle of the references.
- Closure Capture Lists: Use capture lists in closures to break strong reference cycles.
- Instruments Profiling: Regularly profile your application using Instruments. Make it an integral part of your development workflow.
Conclusion
Swift’s memory management, powered by ARC, is efficient, but it’s essential to understand its workings to avoid pitfalls. By adhering to best practices and continuously profiling your app, you can ensure optimal resource usage, leading to better-performing iOS apps. If you’re looking to elevate your projects, consider the option to hire Swift developers for expertise and guidance.
Table of Contents
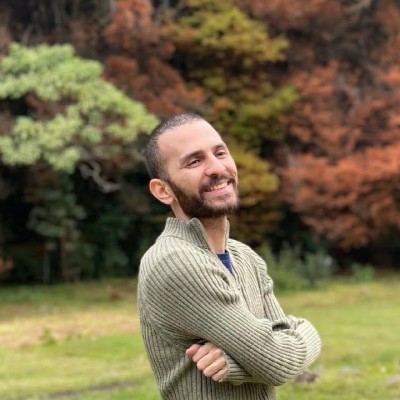
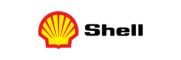