How do I work with method swizzling in Swift?
Method swizzling is a powerful and somewhat advanced technique in Swift and Objective-C that allows you to exchange or modify the implementation of methods at runtime. While it can be handy in certain situations, it should be used cautiously because misuse can lead to unexpected behavior and hard-to-debug issues.
Here are the steps to work with method swizzling in Swift:
- Import Objective-C Runtime: Method swizzling relies on Objective-C runtime features, so you need to import the Objective-C runtime library by adding `#import <objc/runtime.h>` at the top of your Swift file. This allows you to access runtime functions and types.
- Create Extension: To swizzle methods, you typically create an extension on the class you want to modify. Define a new method with the desired behavior.
- Exchange Methods: Use the `method_exchangeImplementations` function from the Objective-C runtime to exchange the implementations of the original method and your custom method. This effectively replaces the original method’s behavior with your custom implementation.
Here’s a simplified example of method swizzling in Swift:
```swift import Foundation import ObjectiveC extension UIViewController { @objc func customViewDidLoad() { // Your custom implementation here print("View did load with method swizzling!") // Call the original method (swizzled method) self.customViewDidLoad() } static func swizzleViewDidLoad() { let originalSelector = #selector(UIViewController.viewDidLoad) let swizzledSelector = #selector(UIViewController.customViewDidLoad) let originalMethod = class_getInstanceMethod(UIViewController.self, originalSelector) let swizzledMethod = class_getInstanceMethod(UIViewController.self, swizzledSelector) if let originalMethod = originalMethod, let swizzledMethod = swizzledMethod { method_exchangeImplementations(originalMethod, swizzledMethod) } } } ```
In this example, we’ve created an extension on `UIViewController` to swizzle the `viewDidLoad` method. We define a custom method `customViewDidLoad` and then use `method_exchangeImplementations` to swap the implementations.
To enable swizzling, you can call `UIViewController.swizzleViewDidLoad()` in your app’s initialization code (e.g., in your AppDelegate). This will ensure that the custom behavior is invoked when any view controller’s `viewDidLoad` is called.
It’s crucial to be cautious when using method swizzling, as it can introduce subtle bugs and make code harder to maintain. Swizzle only when necessary and document it thoroughly to ensure that the behavior is clear to other developers working on the project.
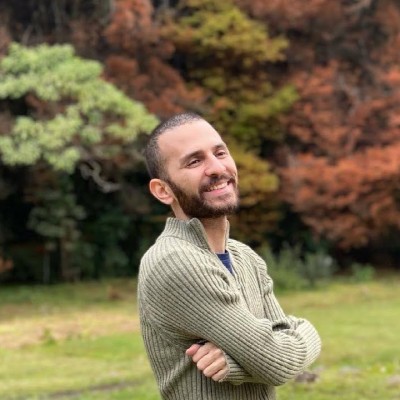
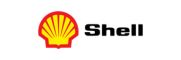