Elevate Your iOS Development Skills with Multithreading in Swift
As application development becomes more complex and data-driven, efficiency and speed are paramount. Swift, Apple’s powerful and intuitive programming language – favored by many companies looking to hire Swift developers – facilitates this through multithreading. Multithreading is a powerful tool that enables the execution of multiple processes or threads concurrently. In the realm of iOS development, multithreading can dramatically boost your application’s performance by efficiently managing CPU resources. This is a key reason why businesses opt to hire Swift developers for their projects. In this blog post, we will delve into the concepts of multithreading in Swift and show how you, or the Swift developers you hire, can use it to optimize your iOS applications with some practical examples.
Table of Contents
1. Understanding Threads
Before diving into multithreading, it is crucial to grasp what a thread is. A thread is the smallest unit of execution within a process. In simpler terms, when you launch an application, the operating system creates a process that contains one or more threads. Each thread can run simultaneously, carrying out different tasks at the same time.
However, we must balance this power with responsibility. Poorly managed threads can lead to issues like race conditions, deadlocks, and thread explosion. Hence, effective thread management is vital for maximizing performance.
2. Multithreading and Concurrency in Swift
Swift provides several high-level abstractions for multithreading and concurrency, such as Dispatch Queues, Operation Queues, and Swift 5.5’s new async/await syntax.
2.1. Dispatch Queues
A Dispatch Queue is a task queue that manages the execution of tasks asynchronously or synchronously on your app’s threads. Dispatch Queues are part of the Grand Central Dispatch (GCD), a low-level API developed by Apple for managing concurrent operations.
There are two types of Dispatch Queues:
- Serial Dispatch Queue: Executes tasks one at a time in the order they were added.
- Concurrent Dispatch Queue: Allows multiple tasks to run concurrently, but doesn’t guarantee the order of completion.
Example
Consider a scenario where we want to download some images and display them on the screen. We can perform this operation asynchronously using Dispatch Queues:
```swift // Create a Dispatch Queue let queue = DispatchQueue(label: "com.example.imageDownloader", attributes: .concurrent) // Add tasks to the queue queue.async { let imageUrl = URL(string: "https://example.com/image1.jpg")! let imageData = try! Data(contentsOf: imageUrl) DispatchQueue.main.async { // Update UI on the main thread imageView1.image = UIImage(data: imageData) } } queue.async { let imageUrl = URL(string: "https://example.com/image2.jpg")! let imageData = try! Data(contentsOf: imageUrl) DispatchQueue.main.async { // Update UI on the main thread imageView2.image = UIImage(data: imageData) } } ```
In this example, we created a concurrent Dispatch Queue that downloads images in the background. We then update the UI on the main thread since UI updates should always be performed on the main thread to avoid UI inconsistency and glitches.
2.2. Operation Queues
Operation Queues are a higher-level abstraction over Dispatch Queues, offering additional functionalities. They allow for task cancellation, setting task dependencies, observing task state, and controlling execution priority.
Example
Assume we have three tasks, TaskA, TaskB, and TaskC. We want TaskA and TaskB to run concurrently, but TaskC should only run after TaskA and TaskB are complete.
```swift let operationQueue = OperationQueue() let operationA = BlockOperation { // Execute TaskA } let operationB = BlockOperation { // Execute TaskB } let operationC = BlockOperation { // Execute TaskC } // Set dependencies operationC.addDependency(operationA) operationC.addDependency(operationB) // Add operations to the queue operationQueue.addOperations([operationA, operationB, operationC], waitUntilFinished: false) ```
In this example, the `addDependency(_:)` method ensures that operationC doesn’t execute until operationA and operationB complete.
2.3. Async/Await in Swift 5.5
Swift 5.5 introduces a new way to handle asynchronous programming with the async/await syntax. It allows you to write asynchronous code almost as if it were synchronous, making it much easier to understand and maintain.
Example
Let’s rewrite the image download example using Swift 5.5’s async/await:
```swift // Create a new async function func downloadImage(from url: String) async throws -> UIImage { let imageUrl = URL(string: url)! let imageData = try Data(contentsOf: imageUrl) return UIImage(data: imageData)! } async { do { let image1 = try await downloadImage(from: "https://example.com/image1.jpg") let image2 = try await downloadImage(from: "https://example.com/image2.jpg") // Update UI on the main thread DispatchQueue.main.async { imageView1.image = image1 imageView2.image = image2 } } catch { // Handle error print(error) } } ```
In this example, `downloadImage(from:)` is an asynchronous function that downloads an image from a URL and returns a UIImage. The `async` block allows us to use the `await` keyword to wait for the `downloadImage(from:)` function to complete before proceeding, improving code readability significantly.
Conclusion
Multithreading is a potent tool in iOS development, significantly enhancing your app’s performance by efficiently managing CPU resources. Swift, the language of choice for many professional Swift developers for hire, offers several high-level abstractions for handling multithreading and concurrency, like Dispatch Queues, Operation Queues, and the newly introduced async/await syntax in Swift 5.5. By understanding and applying these concepts, you can optimize your iOS applications, providing a smoother user experience.
Remember, while multithreading offers several benefits, it’s also crucial to manage threads effectively to avoid potential issues. This is a skill you should seek when you hire Swift developers. Always ensure that UI updates are done on the main thread, avoid simultaneous access to shared resources, and use high-level APIs like Operation Queues or async/await whenever possible to simplify thread management. The right application of these skills can make your coding process efficient and fruitful. Happy coding!
Table of Contents
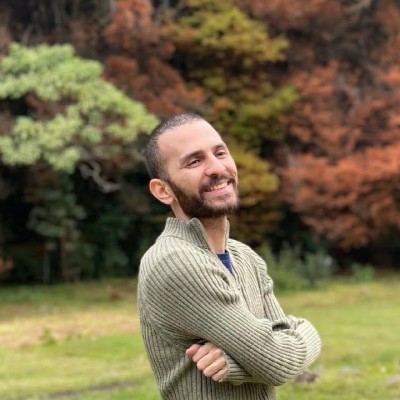
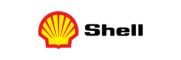