Swift and Natural Language Processing: Analyzing Text in iOS Apps
Understanding Natural Language Processing in iOS
Natural Language Processing (NLP) involves the interaction between computers and human language. It allows apps to understand, interpret, and generate human language in a valuable way. In the context of iOS apps, NLP can be used for tasks like sentiment analysis, language detection, and text classification, enhancing user interactions and delivering personalized experiences.
Using Swift for Text Analysis
Swift, with its powerful libraries and integration with Apple’s Core ML and Natural Language frameworks, is an excellent language for implementing NLP in iOS apps. Below are some key aspects and code examples demonstrating how Swift can be employed for text analysis.
1. Text Tokenization
Tokenization is the process of splitting text into individual components, such as words or sentences. This is often the first step in any NLP task.
Example: Tokenizing Text in Swift
You can use the `NLTokenizer` class from Apple’s Natural Language framework to tokenize text into words or sentences.
```swift import NaturalLanguage let text = "Swift is a powerful language for NLP. It enables sophisticated text analysis." let tokenizer = NLTokenizer(unit: .word) tokenizer.string = text tokenizer.enumerateTokens(in: text.startIndex..<text.endIndex) { tokenRange, _ in let token = text[tokenRange] print(token) return true } ```
2. Sentiment Analysis
Sentiment analysis helps in determining the sentiment expressed in a piece of text, such as whether the text is positive, negative, or neutral.
Example: Performing Sentiment Analysis
The `NLSentimentClassifier` can be used to classify the sentiment of a given text.
```swift import NaturalLanguage let sentimentPredictor = NLSentimentClassifier() let text = "I love using Swift for developing iOS apps!" if let sentiment = sentimentPredictor.predictSentiment(for: text) { print("The sentiment is \(sentiment)") } ```
3. Language Detection
Detecting the language of a text is a fundamental NLP task, especially in multilingual applications.
Example: Language Detection Using Swift
You can use the `NLLanguageRecognizer` to detect the language of a given text.
```swift import NaturalLanguage let text = "Bonjour tout le monde" let recognizer = NLLanguageRecognizer() recognizer.processString(text) if let language = recognizer.dominantLanguage { print("The language is \(language.rawValue)") } ```
4. Named Entity Recognition (NER)
Named Entity Recognition identifies entities like names, places, and dates in text.
Example: Performing NER in Swift
Using the `NLTagger`, you can extract entities from text.
```swift import NaturalLanguage let text = "Apple was founded by Steve Jobs in Cupertino." let tagger = NLTagger(tagSchemes: [.nameType]) tagger.string = text let options: NLTagger.Options = [.omitWhitespace, .omitPunctuation] tagger.enumerateTags(in: text.startIndex..<text.endIndex, unit: .word, scheme: .nameType, options: options) { tag, tokenRange in if let tag = tag { print("\(text[tokenRange]): \(tag.rawValue)") } return true } ```
5. Text Classification
Text classification is used to categorize text into predefined categories, such as spam detection or topic classification.
Example: Text Classification with Core ML
You can train a custom Core ML model to classify text and use it in Swift.
```swift import CoreML import NaturalLanguage let textClassifier = try NLModel(mlModel: MyTextClassifier().model) let text = "This is an amazing feature!" let category = textClassifier.predictedLabel(for: text) print("The text is classified as: \(category ?? "unknown")") ```
Conclusion
Swift, coupled with Apple’s NLP and Core ML frameworks, provides powerful tools for implementing natural language processing in iOS apps. From tokenization and sentiment analysis to language detection and named entity recognition, Swift enables developers to create sophisticated and intelligent text analysis features. By leveraging these capabilities, iOS apps can deliver more personalized and engaging experiences for users.
Further Reading:
Table of Contents
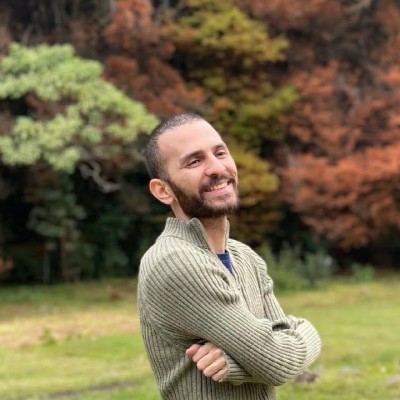
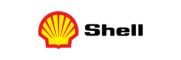