Swift Networking: Communicating with Web Services in iOS Development
In today’s interconnected world, communicating with web services is an essential aspect of iOS app development. Whether you’re fetching data from a remote server, uploading files, or interacting with APIs, a robust networking framework is crucial. Swift Networking provides developers with a powerful toolset to seamlessly integrate web services into their iOS applications. In this blog, we’ll explore the essentials of Swift Networking, including code samples and best practices, to help you create efficient and reliable networking solutions.
Why Swift Networking?
Swift Networking offers a robust and flexible framework for communicating with web services in iOS development. It provides an easy-to-use interface, asynchronous operations, and various functionalities to simplify the process of making HTTP requests, handling responses, and managing networking tasks. Whether you’re working with RESTful APIs, SOAP services, or other web-based communication protocols, Swift Networking streamlines the integration and enhances the overall performance of your iOS applications.
Setting Up Swift Networking
To get started with Swift Networking, you’ll need to add the necessary dependencies to your project. The most common way is by using CocoaPods, a dependency manager for Swift and Objective-C projects. Open your terminal and navigate to your project directory. Create a file named ‘Podfile’ and add the following lines:
ruby platform :ios, '13.0' target 'YourApp' do use_frameworks! pod 'SwiftNetworking' end
Save the file and run the following command in the terminal:
pod install
This will install the Swift Networking framework into your project. Now you’re ready to start integrating web services into your iOS app.
Making HTTP Requests
1. GET Requests:
Making a GET request using Swift Networking is straightforward. Here’s an example:
swift import SwiftNetworking let url = URL(string: "https://api.example.com/posts")! Networking.get(url: url) { result in switch result { case .success(let response): print(response.data) // Access the response data case .failure(let error): print(error.localizedDescription) // Handle the error } }
In this example, we create a URL object and use the get(url:completion:) function from Swift Networking to make a GET request. The result is then handled in a closure, where we can access the response data or handle any errors that occurred.
2. POST Requests:
Performing a POST request requires sending data to the server. Swift Networking simplifies this process with its post(url:parameters:completion:) function. Here’s an example:
swift let url = URL(string: "https://api.example.com/posts")! let parameters = ["title": "New Post", "body": "Lorem ipsum"] Networking.post(url: url, parameters: parameters) { result in // Handle the result }
In this example, we specify the URL and parameters as a dictionary. Swift Networking takes care of serializing the parameters into the appropriate format, such as JSON, and sends the POST request to the server.
3. Handling Responses:
When making HTTP requests, it’s essential to handle the response appropriately. Swift Networking provides a flexible response object that contains information such as the status code, headers, and data. Here’s an example of handling a response:
swift Networking.get(url: url) { result in switch result { case .success(let response): print(response.statusCode) // Access the status code print(response.headers) // Access the headers print(response.data) // Access the response data case .failure(let error): print(error.localizedDescription) // Handle the error } }
By accessing the statusCode, headers, and data properties of the response object, you can extract the necessary information and use it within your app.
Error Handling and Retry Mechanisms
Handling errors and implementing retry mechanisms are crucial for a robust networking solution. Swift Networking simplifies error handling by providing a dedicated NetworkingError enum. You can handle errors using pattern matching, as shown in the following example:
swift Networking.get(url: url) { result in switch result { case .success(let response): print(response.data) case .failure(let error): if let networkingError = error as? NetworkingError { switch networkingError { case .networkFailure(let underlyingError): print("Network failure: \(underlyingError.localizedDescription)") case .requestFailure(let statusCode): print("Request failure: \(statusCode)") case .parsingFailure(let underlyingError): print("Parsing failure: \(underlyingError.localizedDescription)") } } else { print(error.localizedDescription) } } }
By matching against the NetworkingError cases, you can handle specific types of errors differently, such as network failures, request failures (e.g., invalid status codes), or parsing failures.
Additionally, Swift Networking provides utilities for implementing retry mechanisms, allowing you to automatically retry failed requests based on configurable conditions, such as network availability or specific error types.
Authentication and Authorization
Many web services require authentication and authorization. Swift Networking provides mechanisms to handle these scenarios seamlessly. You can add headers to your requests, such as an authentication token, using the headers parameter in the networking functions.
swift let headers = ["Authorization": "Bearer <your_token>"] Networking.get(url: url, headers: headers) { result in // Handle the result }
By including the appropriate headers, you can authenticate your requests and interact with authorized endpoints.
Uploading and Downloading Files
Swift Networking also supports file upload and download functionalities. To upload a file, you can use the uploadFile(url:fileURL:parameters:completion:) function. Here’s an example:
swift let fileURL = URL(fileURLWithPath: "path/to/file") Networking.uploadFile(url: url, fileURL: fileURL, parameters: parameters) { result in // Handle the result }
For downloading files, you can utilize the downloadFile(url:destinationURL:completion:) function. This allows you to specify the destination URL where the downloaded file should be saved.
Handling Background Tasks:
In iOS, it’s common to perform network operations in the background to avoid blocking the main thread. Swift Networking provides support for background tasks, ensuring your app can continue communicating with web services even when it’s in the background. You can use the beginBackgroundTask() and endBackgroundTask(_:) methods to manage background tasks effectively.
Best Practices for Swift Networking
When working with Swift Networking or any networking framework, it’s essential to follow best practices for optimal performance and reliability. Here are a few tips to consider:
- Implement proper error handling and retry mechanisms.
- Use background tasks for long-running operations.
- Securely handle sensitive data and implement appropriate authentication measures.
- Implement appropriate caching mechanisms to improve performance.
- Monitor and optimize network requests to minimize data usage and latency.
Conclusion
In this blog post, we explored the essentials of Swift Networking for communicating with web services in iOS development. We covered making HTTP requests, handling responses, error handling, authentication, file uploading and downloading, background tasks, and best practices. By leveraging Swift Networking, you can create seamless and efficient networking solutions in your iOS applications, providing users with reliable and responsive experiences.
Table of Contents
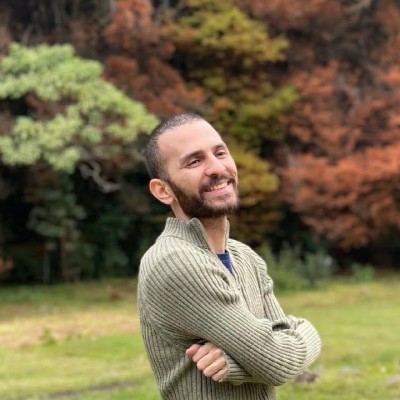
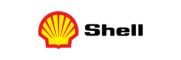