Swift Q & A
What is a nil in Swift?
In Swift, “nil” represents the absence of a value. It’s used to indicate that a variable or constant doesn’t currently hold any meaningful data. Nil is not the same as an empty string, zero, or any default value; it explicitly means “no value.”
Here are some key points about nil in Swift:
- Optional Values: Nil is closely associated with optionals, which are a fundamental concept in Swift. Optionals allow variables or constants to hold either a value or nil, indicating that the value may be missing or undefined.
- Type Safety: Swift is a statically-typed language, and nil is type-safe. This means that you can’t assign nil to a variable or constant that doesn’t explicitly declare itself as an optional. For example, you can’t assign nil to a non-optional `Int` variable.
- Handling Optionals: To work with optionals that may contain nil, Swift provides various mechanisms like optional binding with `if let` or `guard let` statements, force unwrapping with `!`, and optional chaining. These tools help ensure that you handle nil values safely in your code, reducing the risk of runtime crashes.
- Common Use Cases: Nil is often used to represent situations where data may not be available or hasn’t been initialized. For example, if you have an optional variable representing a user’s middle name, it may be nil if the user doesn’t have a middle name. This is preferable to using a default value that might not accurately represent the absence of data.
- Checking for Nil: You can check if an optional variable contains nil using conditional statements. For example:
```swift
if myOptionalVariable == nil {
// Handle the case when myOptionalVariable is nil
}
```
```swift
if myOptionalVariable == nil {
// Handle the case when myOptionalVariable is nil
}
```
```swift if myOptionalVariable == nil { // Handle the case when myOptionalVariable is nil } ```
Nil plays a crucial role in Swift’s safety features, allowing developers to handle situations where data might be missing or undefined without causing runtime errors. By embracing nil and optionals, Swift encourages robust and reliable code that gracefully handles the absence of values.
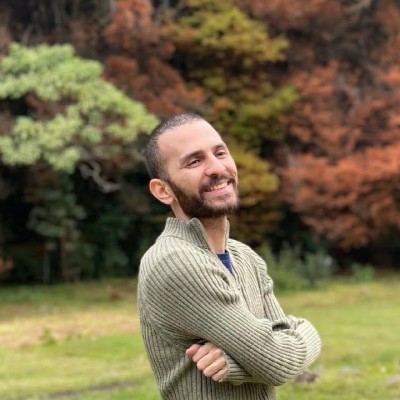
Previously at
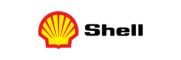
Experienced iOS Engineer with 7+ years mastering Swift. Created fintech solutions, enhanced biopharma apps, and transformed retail experiences.