What is option chaining in Swift?
Option chaining in Swift is a feature that provides a concise and safe way to work with properties, methods, and subscripts of optional values. It allows you to chain multiple optional accesses together in a single statement without the need for nested if-let or guard statements. Option chaining is particularly useful when you’re dealing with complex data structures or when you’re uncertain if a value is present or nil.
Here’s how option chaining works:
- Accessing Properties: You can use optional chaining to access properties of an optional value. If the optional value is nil, the entire chain evaluates to nil without causing a runtime error.
```swift class Person { var address: Address? } struct Address { var street: String } let person: Person? = ... // An optional Person let street = person?.address?.street // 'street' is an optional String ```
In this example, if `person` or `address` is nil, `street` will be nil without any crashes.
- Calling Methods: You can also call methods on optional values using option chaining. If the optional value is nil, the method call is skipped, and the result is nil.
```swift class Engine { func start() { // Start the engine } } var car: Engine? = ... // An optional Engine car?.start() // Calls 'start()' only if 'car' is not nil ```
- Accessing Subscripts: Option chaining can be used to access subscripts of optional values, such as dictionaries or arrays.
```swift var scores: [String: Int]? = ["Alice": 95, "Bob": 89] let aliceScore = scores?["Alice"] // 'aliceScore' is an optional Int ```
Option chaining simplifies code by allowing you to perform a series of operations on optional values in a clean and readable manner. It helps avoid the need for extensive unwrapping and conditional checks, leading to more concise and safer code. However, it’s essential to understand that option chaining results in optional values, so you may need to unwrap them using if-let or guard statements if you want to work with the underlying non-optional values.
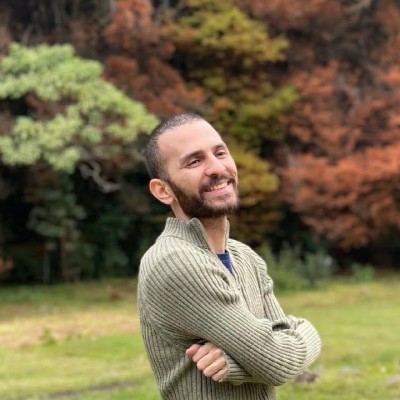
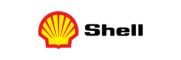