What is an optional in Swift?
In Swift, an optional is a unique feature that allows variables or constants to have either a value or no value at all. It’s a powerful tool for handling situations where a value might be missing, and it helps in preventing runtime crashes due to nil (null) values. Optionals are represented using the `Optional` type, which is a generic enumeration with two possible cases: `.some(wrapped)` for a value and `.none` for no value.
Here’s how optionals work:
- Declaration: To declare an optional variable or constant, you append a question mark `?` after the data type. For example:
```swift var age: Int? // An optional integer that can have a value or be nil var name: String? // An optional string ```
- Assigning a Value: You can assign a value to an optional using the `.some` case. For example:
```swift age = 25 ```
- Assigning nil: To represent the absence of a value, you can assign nil to an optional. For example:
```swift age = nil ```
- Unwrapping: To access the value of an optional, you need to unwrap it. You can do this using optional binding with `if let` or `guard let` statements, or by force unwrapping using `!`. However, using force unwrapping should be done with caution, as it can lead to runtime crashes if the optional is nil.
```swift if let validAge = age { // The optional has a value, and it's safe to use validAge here } else { // The optional is nil } ```
- Optional Chaining: You can use optional chaining to access properties, methods, and subscripts of an optional that might be nil. This enables you to work with optionals in a safe and concise manner.
```swift let streetName = address?.street?.name ```
- Implicitly Unwrapped Optionals: You can declare an implicitly unwrapped optional using `!` after the data type. These optionals are automatically unwrapped when accessed, assuming they have a value. They are typically used when you know an optional will always have a value after its initial assignment.
```swift var phoneNumber: String! = "123-456-7890" let length = phoneNumber.count // No need to unwrap phoneNumber here ```
In summary, optionals in Swift provide a robust way to handle values that may be missing or unknown, reducing the risk of runtime crashes due to nil values. They are a fundamental part of Swift’s safety features, promoting safer and more reliable code. Developers use optionals extensively in Swift to handle a wide range of scenarios where values may or may not be present.
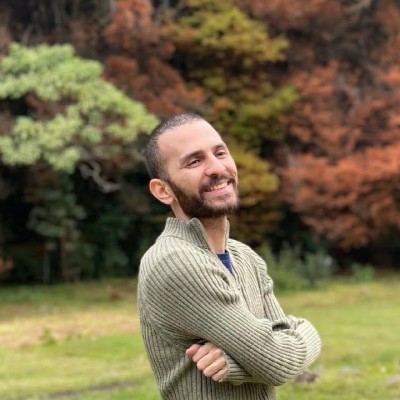
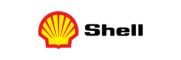