Swift Performance Tuning: Optimizing Speed and Memory Usage in iOS
Understanding Swift Performance Tuning
Performance tuning in iOS development involves optimizing applications to run faster and use memory more efficiently. This is crucial for providing a smooth user experience and ensuring that apps perform well across a range of devices. In Swift, there are several techniques and best practices to achieve optimal performance.
Techniques for Speed Optimization
1. Profiling Your Application
Before optimizing, it’s essential to understand where your application spends the most time. Xcode’s Instruments tool can help identify performance bottlenecks and areas for improvement.
Example: Profiling with Instruments
Use Instruments to analyze your app’s CPU and memory usage, network activity, and more. This will guide you in identifying areas that require optimization.
2. Efficient Data Structures
Choosing the right data structures can significantly impact performance. For instance, using arrays or dictionaries appropriately can reduce computation time.
Example: Using Dictionaries for Faster Lookups
```swift var userCache: [String: User] = [:] func getUser(byID id: String) -> User? { return userCache[id] } ```
Using a dictionary allows O(1) time complexity for lookups compared to O(n) with an array.
3. Avoiding Expensive Operations
Be mindful of operations that are costly in terms of performance, such as complex calculations or frequent I/O operations. Optimize these by performing them asynchronously or caching results.
Example: Asynchronous Processing
```swift DispatchQueue.global(qos: .background).async { let result = performComplexCalculation() DispatchQueue.main.async { updateUI(with: result) } } ```
4. Lazy Loading and Deferred Execution
Load resources and perform actions only when needed to improve initial load times and overall performance.
Example: Lazy Initialization
```swift lazy var expensiveResource: ExpensiveResource = { return ExpensiveResource() }() ```
Techniques for Memory Optimization
1. Memory Management with ARC
Automatic Reference Counting (ARC) in Swift helps manage memory efficiently. Ensure you understand strong, weak, and unowned references to avoid retain cycles and memory leaks.
Example: Using Weak References
```swift class ViewController: UIViewController { weak var delegate: SomeDelegate? } ```
2. Reducing Memory Footprint
Optimize memory usage by avoiding large objects and images when not necessary. Use image compression and efficient data formats.
Example: Compressing Images
```swift func compressImage(_ image: UIImage) -> Data? { return image.jpegData(compressionQuality: 0.5) } ```
3. Optimizing Collection Usage
Large collections can consume significant memory. Use more memory-efficient collections or consider alternatives like caching.
Example: Using NSCache
```swift let imageCache = NSCache<NSString, UIImage>() func cacheImage(_ image: UIImage, forKey key: String) { imageCache.setObject(image, forKey: key as NSString) } ```
4. Profiling and Analyzing Memory Usage
Use Xcode’s Memory Graph Debugger to identify and fix memory issues, such as leaks and excessive allocations.
Example: Memory Leak Detection
Run the Memory Graph Debugger to visualize object relationships and identify potential leaks.
Conclusion
Optimizing speed and memory usage in iOS applications using Swift involves a combination of profiling, efficient coding practices, and leveraging the right tools. By applying these techniques, you can enhance the performance and efficiency of your iOS apps, leading to a better user experience.
Further Reading:
Table of Contents
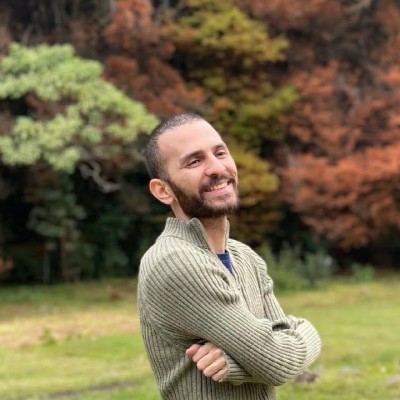
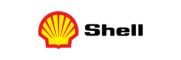