What is protocol-oriented programming in Swift?
Protocol-oriented programming (POP) is a programming paradigm in Swift that emphasizes the use of protocols to define and structure your code. It is an alternative to traditional object-oriented programming (OOP) and is particularly well-suited for Swift’s modern language features. In POP, protocols play a central role in defining blueprints for behavior, allowing you to create highly reusable and composable code.
Here are key concepts and principles of protocol-oriented programming in Swift:
- Protocols Define Behavior: In POP, protocols are used to define interfaces or contracts that specify the behavior that types (classes, structs, enums) must adhere to. Protocols declare methods, properties, and associated types that conforming types must implement.
- Composition Over Inheritance: POP encourages composition of behavior over inheritance. Instead of creating deep inheritance hierarchies, you define protocols for specific behaviors and have types adopt multiple protocols to gain the desired functionality. This promotes code reuse and flexibility.
- Protocol Extensions: Protocol extensions allow you to provide default implementations for methods and properties defined in protocols. Conforming types can choose to use these defaults or provide their own custom implementations.
- Generics: Swift’s generics system complements POP, enabling you to write highly flexible and reusable code by defining protocols that work with generic types.
- Conformance: Types can conform to one or multiple protocols, which means they promise to implement the required behavior defined by those protocols. This allows you to create highly modular and interchangeable components.
- Decoupling: POP promotes loose coupling between components, making your codebase more maintainable and testable. It reduces dependencies on concrete types and encourages dependency injection.
- Protocol-Oriented Design: POP encourages a design philosophy where you identify and define protocols for the distinct behaviors and functionalities of your application. This approach often leads to cleaner and more modular code.
Here’s a simplified example of protocol-oriented programming in Swift:
```swift protocol Drawable { func draw() } protocol Animatable { func animate() } struct Circle: Drawable, Animatable { func draw() { // Drawing code for a circle } func animate() { // Animation code for a circle } } ```
In this example, we have two protocols, `Drawable` and `Animatable`, defining behaviors. The `Circle` struct conforms to both protocols, implementing the `draw()` and `animate()` methods to specify its behavior.
In summary, protocol-oriented programming in Swift is a powerful approach to building modular, reusable, and flexible code. By using protocols to define behavior and composing types with multiple protocols, you can create robust and maintainable software that adapts to changing requirements and promotes code reusability.
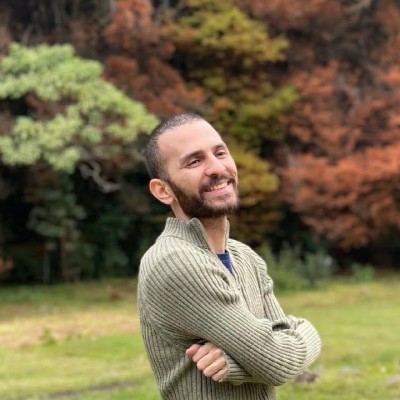
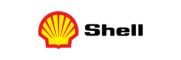