Boost Your iOS App’s Reach with Swift-Based Push Notifications
Push notifications are one of the most effective ways to engage with users of your mobile app. When utilized correctly, they can greatly improve user retention, increase user interaction, and boost app monetization. If you’re looking to enhance your app further, consider hiring Swift developers for expert insights. This article provides an overview of how to implement push notifications in your iOS apps using Swift, as well as some best practices for creating engaging notifications.
Table of Contents
1. What are Push Notifications?
Push notifications are messages that are sent directly to a user’s mobile device, even if the app is not running in the foreground. They can be used to notify users of new content, remind them of important tasks, or simply engage them with your app. Examples include:
– E-commerce Apps: “Your order has shipped!”
– Social Media Apps: “John just liked your photo!”
– News Apps: “Breaking news: Major earthquake in California!”
– Gaming Apps: “Your energy is now full. Play now!”
2. Setting up Push Notifications in iOS with Swift
Setting up push notifications for iOS involves several steps:
- Configuration in the Apple Developer Portal:
– Register your app.
– Create an App ID with push notifications enabled.
– Generate an Apple Push Notification Service (APNs) Key.
- Configuration in Xcode:
– Enable push notifications in your app capabilities.
– Request user permission to receive notifications.
– Register for remote notifications.
- Handling Notifications:
– Receive and process notifications in your app.
– Display the notification content.
- Server-Side Configuration:
– Send notifications from your server to APNs, which will then deliver them to users.
Let’s walk through the basics of each step:
2.1. Configuration in the Apple Developer Portal
– Register Your App:
Visit the Apple Developer Portal, navigate to Certificates, IDs & Profiles, and add a new App ID. Ensure you enable push notifications for this App ID.
– Generate an APNs Key:
Create a new Key under the “Keys” section. Make sure “Apple Push Notifications service (APNs)” is checked. Download the key and safely store it – you’ll need it for your server-side setup.
2.2. Configuration in Xcode
– Enable Push Notifications:
In your Xcode project, go to the ‘Capabilities’ tab and switch on ‘Push Notifications’.
– Request User Permission:
Before sending push notifications, you need to request permission from the user. Use the `UNUserNotificationCenter` to achieve this:
```swift import UserNotifications let center = UNUserNotificationCenter.current() center.requestAuthorization(options: [.alert, .sound, .badge]) { (granted, error) in // Handle the user's decision here } ```
– Register for Remote Notifications:
Once you have the user’s permission, register the device to receive remote notifications:
```swift UIApplication.shared.registerForRemoteNotifications() ```
3. Handling Notifications
When your app receives a notification, you’ll need to handle and display its content. You can do this using various delegate methods provided by `UNUserNotificationCenter`.
For instance, to handle a notification when your app is in the foreground:
```swift func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) { completionHandler([.alert, .sound, .badge]) } ```
4. Server-Side Configuration
The final piece of the puzzle is server-side. Using your APNs key, send push notifications to APNs, specifying the device tokens of the intended recipients.
There are various server-side languages and frameworks you can use for this, like Node.js with the `node-apn` library, or Python with `pyapns2`.
5. Best Practices for Engaging Notifications
- Relevance: Ensure that your push notifications are relevant to the user. Irrelevant notifications can be seen as spammy and lead to app deletions.
- Timeliness: Time your notifications correctly. For example, don’t send game invites in the middle of the night.
- Frequency: Avoid bombarding your users with too many notifications. This can lead to notification fatigue.
- Actionable: Make your notifications actionable. A message like “Check out our new items!” is more engaging than a generic “Open our app!”.
Conclusion
Push notifications are a powerful tool in an app developer’s arsenal. By integrating them into your Swift iOS apps, you can provide timely, relevant, and actionable alerts that can significantly improve user engagement. If you’re aiming for perfection, you might want to hire Swift developers to elevate your app’s potential further. With the steps provided above and a focus on user-centric notifications, you’re well on your way to creating an app that users will love to engage with.
Table of Contents
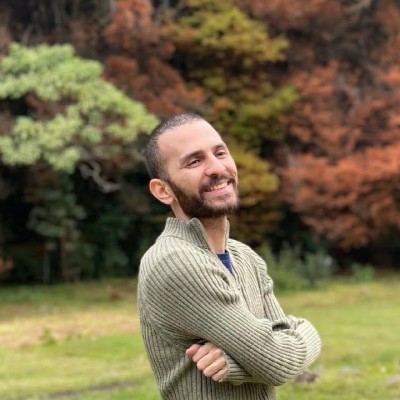
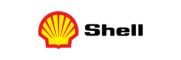