How the Combine Framework is Revolutionizing iOS Development with Reactive Programming
Reactive programming is a programming paradigm centered on data streams and the propagation of change. Instead of the traditional imperative way of programming, in reactive programming, we describe how data flows and transformations occur over time. Apple’s Combine framework, introduced in 2019, is a powerful tool for Swift developers looking to harness the power of reactive programming in their iOS applications. If you’re looking to elevate your projects, it might be the right time to hire Swift developers who are adept at leveraging this framework.
Table of Contents
In this blog post, we’ll delve into the essentials of Combine and provide some real-world examples to showcase its capabilities.
1. The Basics of Combine
The Combine framework offers a standardized interface for processing values over time. There are three fundamental components:
- Publishers – Emit a sequence of values over time.
- Subscribers – Receive and react to the values emitted by publishers.
- Operators – Transform or manipulate values emitted by publishers.
2. Getting Started with Publishers and Subscribers
The first thing you’ll often deal with in Combine is a Publisher. Let’s see how a basic publisher and subscriber interaction works:
```swift import Combine let justPublisher = Just("Hello, Combine!") let subscriber = Subscribers.Sink<String, Never> { completion in switch completion { case .finished: print("Completed!") case .failure(let error): print("Error: \(error)") } } receiveValue: { value in print("Received value: \(value)") } justPublisher.subscribe(subscriber) ```
In this example, `Just` is a publisher that emits a single value and then completes. The `Sink` subscriber receives the value and completion event, printing them to the console.
3. Common Publishers
Combine provides several built-in publishers. Here are a few:
– Just: Emits a single value and then completes.
– Fail: Immediately terminates with an error.
– Empty: Immediately completes without emitting any values.
– Future: Starts a potentially asynchronous computation and can emit a single value or an error.
– PassthroughSubject: A subject that broadcasts values to subscribers.
4. Operators
Operators are methods provided by publishers that transform or manipulate the emitted values. Let’s see a practical example:
```swift import Combine let numbers = [1, 2, 3, 4, 5].publisher numbers .filter { $0 % 2 == 0 } .map { $0 * 2 } .sink(receiveValue: { print($0) }) // Outputs: // 4 // 8 ```
Here, we use the `filter` operator to get even numbers and then the `map` operator to double them.
5. Handling Asynchronous Operations with Combine
Combine shines when handling asynchronous operations. Here’s an example using `Future`:
```swift import Combine func fetchUserId() -> Future<Int, Error> { return Future { promise in DispatchQueue.global().asyncAfter(deadline: .now() + 2) { promise(.success(12345)) } } } fetchUserId() .sink(receiveCompletion: { completion in if case .failure(let error) = completion { print("Error fetching user ID: \(error)") } }, receiveValue: { userId in print("Fetched user ID: \(userId)") }) // Outputs after 2 seconds: // Fetched user ID: 12345 ```
6. Combining Multiple Publishers
A common scenario in app development is needing to combine outputs from multiple publishers. Combine provides operators like `merge`, `combineLatest`, and `zip` for these purposes.
Here’s an example using `combineLatest`:
```swift import Combine let namePublisher = PassthroughSubject<String, Never>() let agePublisher = PassthroughSubject<Int, Never>() namePublisher .combineLatest(agePublisher) .sink { name, age in print("\(name) is \(age) years old.") } namePublisher.send("Alice") agePublisher.send(28) // Outputs: Alice is 28 years old. ```
7. Error Handling
Combine provides operators to handle errors, like `catch` and `retry`. Here’s a simple example using `catch`:
```swift import Combine let failPublisher = Fail(outputType: Int.self, failure: MyError.someError) failPublisher .catch { _ in Just(-1) } // On error, provide a fallback value. .sink(receiveCompletion: { _ in }, receiveValue: { value in print(value) // Outputs: -1 }) ```
Conclusion
Reactive programming with Swift’s Combine framework offers a structured and potent method to manage data flows and asynchronous operations in iOS applications. If you’re considering elevating your app’s capabilities, it might be the right time to hire Swift developers proficient in this domain. While this post has only scratched the surface, it aims to provide a foundational understanding to begin experimenting with reactive paradigms in your upcoming projects. With dedication and practice, the Combine framework can become an indispensable tool in any iOS developer’s arsenal.
Table of Contents
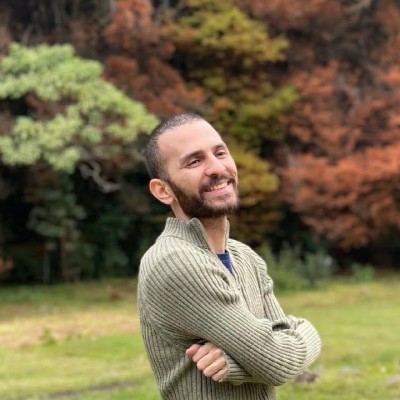
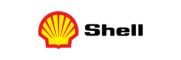