How do I use ‘repeat-while’ loops in Swift?
A ‘repeat-while’ loop in Swift is a control structure that allows you to repeatedly execute a block of code until a specified condition becomes false. It’s similar to a ‘while’ loop, but with one crucial difference: a ‘repeat-while’ loop always executes its block of code at least once, even if the condition is initially false. After the code block executes, it checks the condition, and if it’s still true, the loop continues. This ensures that the loop body is executed at least once, which can be useful in situations where you want to guarantee the execution of a block of code before checking the condition.
Here’s how to use a ‘repeat-while’ loop in Swift:
- Initialization: You declare any necessary variables and initialize them before entering the loop.
- Loop Body: The code inside the ‘repeat-while’ loop’s block gets executed at least once.
- Condition Check: After the loop body executes, the condition is checked. If it’s true, the loop continues; if it’s false, the loop terminates.
Here’s a simple example of a ‘repeat-while’ loop that asks the user for input until they enter a valid number:
```swift var userNumber: Int repeat { print("Enter a number: ") if let input = readLine(), let number = Int(input) { userNumber = number } else { userNumber = -1 } } while userNumber < 0 ```
In this example, the loop body asks the user for a number and attempts to convert their input into an integer. If the input is not a valid number, the ‘userNumber’ variable is set to -1. The loop continues until the user enters a non-negative number.
‘repeat-while’ loops are valuable when you need to ensure that a specific block of code runs at least once, regardless of the initial condition. They are commonly used for input validation and interactive user interfaces, among other scenarios.
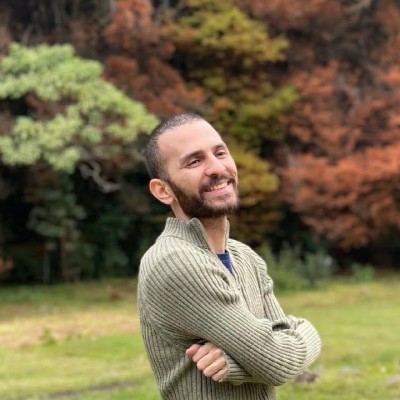
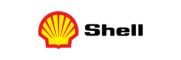