How do I create and use sets in Swift?
Sets in Swift are unordered collections of unique values. They provide a way to store and manipulate data in a way that ensures uniqueness and doesn’t rely on a specific order. Here’s how you can create and use sets in Swift:
Creating Sets:
To create a set in Swift, you use the `Set` type with a specific data type, or Swift can infer the data type based on the values you provide:
```swift var fruits: Set<String> = ["apple", "banana", "orange"] var colors = Set(["red", "green", "blue"]) ```
In these examples, we’ve created sets of strings and allowed Swift to infer the type of the `colors` set.
Adding and Removing Elements:
You can add and remove elements from a set using the `insert` and `remove` methods:
```swift fruits.insert("grape") fruits.remove("banana") ```
These operations maintain the uniqueness property of sets, ensuring that there are no duplicate values.
Accessing and Iterating:
You can access and iterate over the elements in a set using `for-in` loops or methods like `contains` to check for the presence of a specific element:
```swift for fruit in fruits { print(fruit) } if fruits.contains("apple") { print("Found apple!") } ```
Set Operations:
Sets support various set operations like union, intersection, and difference. For example:
```swift let set1: Set<Int> = [1, 2, 3, 4] let set2: Set<Int> = [3, 4, 5, 6] let union = set1.union(set2) // {1, 2, 3, 4, 5, 6} let intersection = set1.intersection(set2) // {3, 4} let difference = set1.subtracting(set2) // {1, 2} ```
These operations allow you to combine and manipulate sets to perform various set-related tasks.
Sets in Swift are highly efficient for checking the presence of unique values and performing set operations. They are a valuable tool when you need to manage collections of data with no duplicates and no specific order requirements.
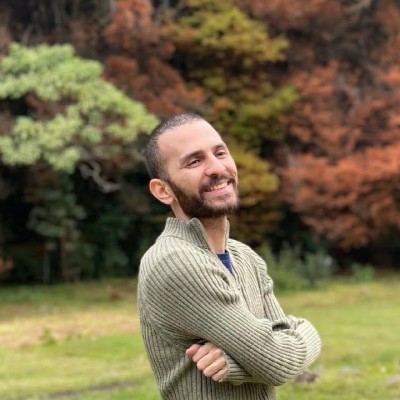
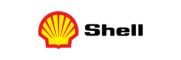