How do I work with sets in Swift?
Working with sets in Swift is essential when you need to manage collections of unique, unordered elements. A set is a data structure that allows you to store distinct values and efficiently perform operations like adding, removing, and checking for membership. In Swift, sets are represented by the `Set` type, which is part of the Swift Standard Library.
Here’s a comprehensive guide on how to work with sets in Swift:
- Creating Sets:
– To create an empty set, you can use the constructor: `var mySet = Set<Type>()`.
– To create a set with initial values, you can provide an array of values: `var colors: Set<String> = [“Red”, “Green”, “Blue”]`.
- Adding and Removing Elements:
– You can add elements using the `insert` method: `mySet.insert(“NewElement”)`.
– To remove elements, use the `remove` method: `mySet.remove(“ElementToRemove”)`.
- Checking Membership:
– You can check if an element is in the set using the `contains` method: `if mySet.contains(“CheckElement”) { /* do something */ }`.
- Iterating Over a Set:
– You can use a `for-in` loop to iterate over the elements: `for element in mySet { /* process element */ }`.
- Set Operations:
– Sets support various set operations like union, intersection, and difference using methods like `union`, `intersection`, and `subtracting`.
```swift let setA: Set<Int> = [1, 2, 3, 4] let setB: Set<Int> = [3, 4, 5, 6] let unionSet = setA.union(setB) // Contains elements from both sets let intersectionSet = setA.intersection(setB) // Contains common elements let diffSet = setA.subtracting(setB) // Contains elements in setA but not in setB ```
- Set Properties:
– You can check the number of elements in a set using `count`.
```swift let count = mySet.count // Number of elements in the set ```
Sets are particularly useful when you need to maintain a collection of unique values, such as keeping track of distinct items in a shopping cart or managing user preferences without duplicates. Understanding how to create, manipulate, and perform operations on sets is a valuable skill for Swift developers.
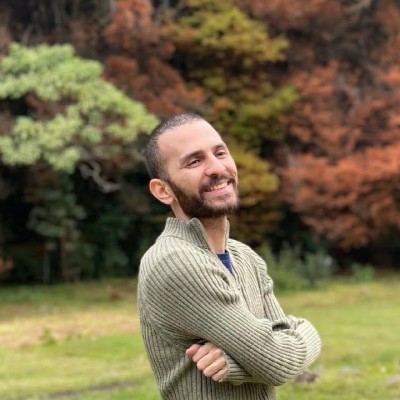
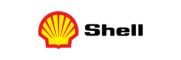