Bring Siri to Your Apps: Integrate SiriKit for Voice-Activated iOS Apps
Siri, Apple’s voice assistant, offers iOS users a hands-free mode to perform a myriad of actions such as making calls, sending text messages, or getting directions. For businesses looking to leverage this technology, the solution is to hire Swift developers who specialize in SiriKit. With their expertise, these professionals can integrate Siri into your applications, instilling a whole new level of interactivity. In this blog post, we’ll explore how hiring Swift developers and harnessing the power of SiriKit can create voice-activated iOS experiences that will transform how users interact with your app.
Table of Contents
1. What is SiriKit?
SiriKit is an iOS framework that allows third-party developers to use Siri’s voice recognition capabilities within their apps. With SiriKit, your apps can interpret voice commands as specific, actionable instructions. It provides domains and intents that categorize different types of user requests. Developers can tailor these intents to their specific app’s functionality, providing a unique, voice-controlled experience for users.
2. Setting Up SiriKit in Your App
To integrate SiriKit into your app, you’ll first need to add the Siri capability in Xcode. Go to your project settings, select “Capabilities,” and turn on Siri. This will add a Siri entitlement to your app.
In addition, you’ll need to add a new ‘Intents Extension’ to your app to handle Siri requests. Navigate to File > New > Target and select ‘Intents Extension’. Remember to enable the ‘Include UI Extension’ option if you want to customize the Siri UI when it displays your app’s responses.
3. Defining Custom Intents
Apple provides a series of built-in intents for common tasks. However, if your app requires unique functionality, you may need to define custom intents. This is done using the ‘Intent Definition File’ in Xcode.
For instance, let’s say we’re creating an app for ordering food. We might create a custom intent called “OrderFoodIntent”. In the Intent Definition File, you’d specify inputs (“pizza”, “sushi”, etc.), and any additional parameters like quantity or delivery time. Xcode generates classes for your custom intents that you’ll use later in your code.
4. Implementing Intent Handlers
Once you’ve defined your custom intents, you’ll need to implement intent handlers in your Intents Extension. These handlers specify what to do when Siri receives a request that matches one of your intents.
For our food ordering app, we’d create a handler for our “OrderFoodIntent”. This handler would verify the order details, place the order, and provide a response to Siri.
```swift import Intents class OrderFoodIntentHandler: NSObject, OrderFoodIntentHandling { func handle(intent: OrderFoodIntent, completion: @escaping (OrderFoodIntentResponse) -> Void) { // Verify the food order details guard let foodItem = intent.food else { completion(OrderFoodIntentResponse(code: .failure, userActivity: nil)) return } // Place the food order // For the sake of this example, we'll assume the order is always successful let response = OrderFoodIntentResponse.success(food: foodItem) completion(response) } } ```
In the `OrderFoodIntentHandler`, we first verify the intent’s parameters. If there’s no food item specified, we return a failure response. Otherwise, we place the food order and return a successful response.
5. Configuring the Intent Handler
After implementing your intent handlers, you need to configure Siri to use them. This is done in the `IntentHandler` class, which serves as a factory for creating the necessary handlers.
```swift import Intents class IntentHandler: INExtension { override func handler(for intent: INIntent) -> Any { // Return the appropriate handler based on the intent type if intent is OrderFoodIntent { return OrderFoodIntentHandler() } return self } } ```
In our case, the `handler(for:)` method returns an instance of `OrderFoodIntentHandler` if the intent is of type `OrderFoodIntent`.
6. User Authorization and Siri Dialog
Finally, you need to handle user authorization and Siri dialog.
To use SiriKit, users need to authorize your app to work with Siri. It’s a good practice to ask for permission when the user first interacts with a Siri-related feature.
```swift INPreferences.requestSiriAuthorization { status in // Handle authorization status } ```
The Siri dialog is the voice and visual interface between the user and Siri. For custom intents, you can specify the dialog prompts and responses in the Intent Definition File.
Returning to our food ordering app, we might define prompts like “What type of food would you like to order?” and responses like “Your order for \(food) has been placed.”
Conclusion
Creating voice-activated experiences for your iOS apps can greatly boost their accessibility and user engagement. This is where the decision to hire Swift developers can make a significant difference. These professionals can utilize SiriKit to infuse your apps with an unmatched level of interactivity. Whether you’re employing built-in intents for common tasks or defining custom intents for unique app features, the potential to enhance your app’s user experience is immense.
While SiriKit may seem complex at first glance, it opens up vast possibilities once you get the hang of it. With the expertise of hired Swift developers, creating interactive, voice-controlled apps becomes a more achievable reality. We hope this article has given you a solid foundation and inspired you to further explore the advantages of incorporating SiriKit into your iOS applications.
Table of Contents
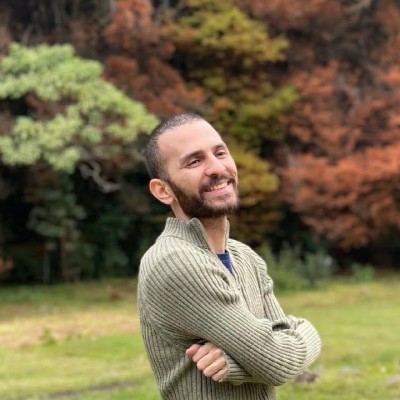
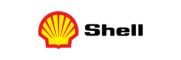