What is a struct in Swift?
In Swift, a struct, short for “structure,” is a user-defined data type that allows you to group together related values and behaviors into a single unit. Structs are similar to classes in many ways, but they have some key differences that make them distinct. Structs are value types, which means they are copied when assigned to a new variable or passed as arguments, rather than being referenced. This can lead to different memory management and behavior compared to classes.
Here are key characteristics and concepts related to structs in Swift:
- Value Types: Structs are value types, which means when you assign a struct instance to another variable or pass it as an argument to a function, a copy of the instance is created. This behavior ensures that structs are immutable by default and that changes to one instance do not affect others.
- Properties: Like classes, structs can have properties that store data specific to an instance. These properties define the attributes or characteristics of the struct.
- Methods: Structs can also contain methods, which are functions associated with the struct. Methods define behaviors or actions that struct instances can perform.
- Initialization: Structs can have initializers, similar to classes, used to set up the initial state of an instance when it’s created.
- No Inheritance: Unlike classes, structs do not support inheritance. You cannot create new structs that inherit properties or methods from existing ones. Each struct is independent.
- Immutability: Structs are often used for creating immutable data models, ensuring that once an instance is created, its properties cannot be changed. This can help prevent unintended side effects in your code.
Here’s a simplified example of a struct in Swift:
```swift struct Point { var x: Double var y: Double init(x: Double, y: Double) { self.x = x self.y = y } func distance(to otherPoint: Point) -> Double { let deltaX = self.x - otherPoint.x let deltaY = self.y - otherPoint.y return sqrt(deltaX * deltaX + deltaY * deltaY) } } ```
In this example, we’ve defined a `Point` struct with properties for `x` and `y` coordinates, an initializer, and a method to calculate the distance between two points.
In summary, structs in Swift are a fundamental data type that allows you to create value-based units for organizing data and behavior. They are particularly useful when you want to ensure immutability and value semantics in your code or when working with simple data structures.
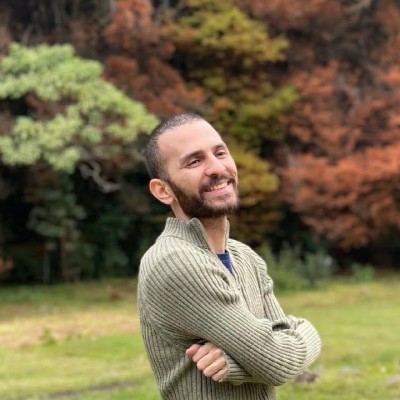
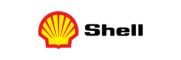