How do I use subscript in Swift?
In Swift, subscripts provide a convenient way to access elements within a collection, such as an array, dictionary, or custom data structure, using a subscript syntax similar to how you access elements by index or key. Subscripts allow you to define custom behavior for retrieving and setting values at a particular index or key within your type. Here’s how you can use subscripts in Swift:
Defining a Subscript:
- Syntax: To define a subscript, you use the ‘subscript’ keyword followed by a set of parentheses with one or more input parameters that specify how to access elements. The return type of the subscript indicates the type of value you can retrieve or set.
```swift subscript(index: Int) -> ElementType { // Define how to get or set the element at 'index' } ```
Accessing Elements:
- Reading Values: You can access elements using subscripts by providing the appropriate index or key inside square brackets. For example, if you have an array-like data structure, you can retrieve elements like this:
```swift let element = myArray[3] // Retrieves the element at index 3 ```
- Setting Values: Subscripts also allow you to set values using the same subscript syntax:
```swift myArray[2] = newValue // Sets the value at index 2 to 'newValue' ```
Multiple Parameters:
- Multiple Parameters: Subscripts can have multiple parameters to enable more complex access patterns. For example, a matrix data structure might use two indices:
```swift subscript(row: Int, column: Int) -> ElementType { // Define how to get or set the element at 'row' and 'column' } ```
Custom Behavior: You can implement custom logic within your subscript to perform operations like bounds checking, data validation, or implementing custom data structures. Subscripts are not limited to arrays and dictionaries; you can create them for any type that needs custom element access.
Subscripts in Swift provide a flexible way to interact with elements in your data structures. They improve code readability and allow you to encapsulate the logic of element access within your types, making your code more expressive and maintainable. Whether you’re working with arrays, dictionaries, or custom collections, subscripts are a powerful tool for customizing element access behavior.
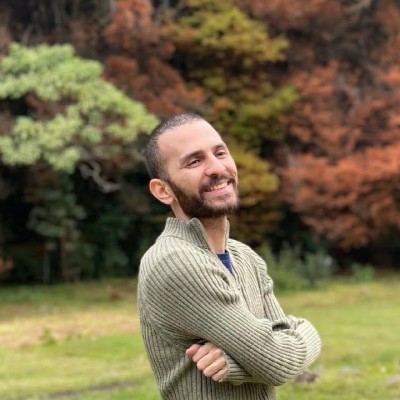
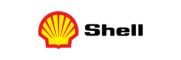